Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial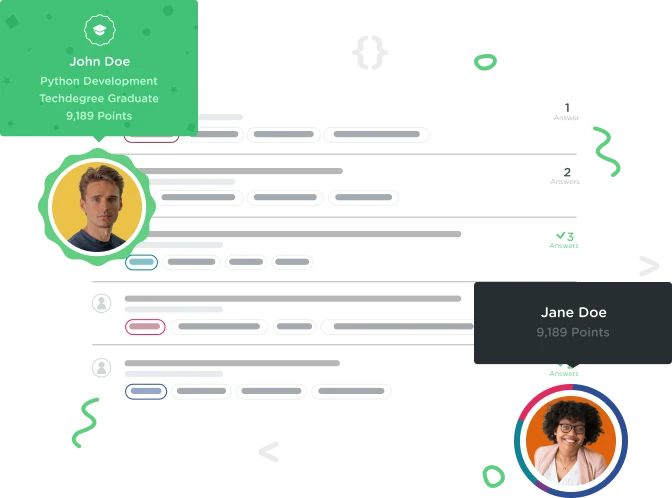

Michael Wadsworth
394 Points"==", "equals", and comparing Strings
I have been previously taught that "==" is only meant to compare equal integers, not Strings. Strings are supposed to be compared with the "equals" command. However, when completing this challenge task, I was able to use both "==" and "equals" to compare a String. Is this an error in the system, or was my teacher incorrect?
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if (firstExample.equals(secondExample))
{
console.printf("first is equal to second");
}
4 Answers

Craig Dennis
Treehouse TeacherI have a workshop releasing tomorrow called "The Thing about Strings" that explains this. I will post it here when it launches. You are right, always compare Strings (and all Objects) with the equals
method.
The reason why this works, is that you can compare object references. If the object reference refers to the same object in memory ==
will return true. Strings are Objects.
But they are different objects you say! Well....the next thing to know is that Strings are immutable, meaning that they cannot be changed. The Java compiler does some work to make sure that string literals, strings that are defined in double quotes, do not produce unneeded memory needs. So what happens is they actually refer to the same object in memory, and therefore ==
is true. Confusing eh?
Good thing there is a workshop coming out ;)
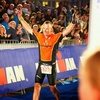
Steve Hunter
57,712 PointsYes, so it does. I was taught the same as you.
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if(firstExample == secondExample){
console.printf("first is equal to second");
}
That passes the task. I didn't think you could compare strings like that ...

Seth Kroger
56,413 Points== and .equals() doesn't do the same thing and only appears to work due to some optimizing Java does with Strings. For example only the if with equals() executes here:
String firstExample = "hello";
String subExample2a = "hel";
String subExample2b = "lo";
String secondExample = subExample2a + subExample2b;
if (firstExample == secondExample) {
System.out.printf("first is equal to second using =="); // Doesn't print
}
if (firstExample.equals(secondExample)) {
System.out.printf("first is equal to second using .equals()");
}
What == does is see if the two variables are referring to the same object, not whether two different objects are equal. Because you have two identical strings being assigned to two variables the Java compiler optimized it to a single String object and made both variables refer it.
Relevant Stackoverflow thread: http://stackoverflow.com/questions/513832/how-do-i-compare-strings-in-java

Craig Dennis
Treehouse TeacherThe Thing About Strings is now live. Totally deals with this weirdness!
Let me know if it clears things up!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsLooking forward to the course ... great stuff!!
:-)
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsSounds great!