Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial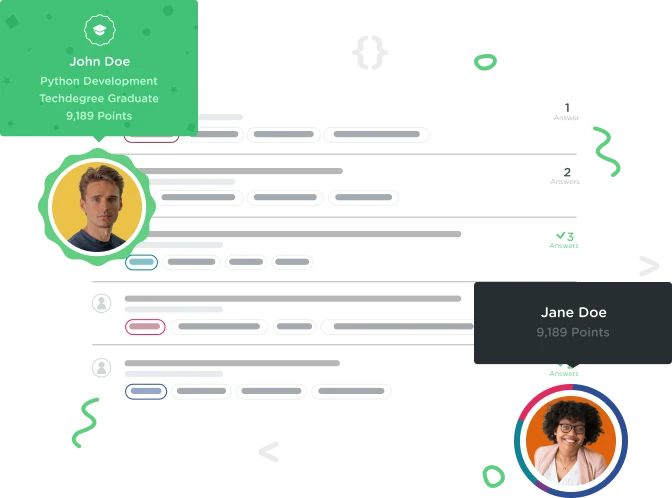

Kyle Baker
1,884 PointsA few questions on Finishing TreeStory challenge
First: when I submit my work it returns with an error that says, "cannot access Main import com.teamtreehouse.Main" and "bad source file" and so forth. Any suggestions on how I can resolve this problem?
Second: When I run the project in IntelliJ it turns the "phrase" into the middle word of the story when it prompts for words. Also, rather than replacing the blanks (__) with the entered words it just replaces the entire middle of the story with the entered words. The story "Ia_boy" with the entered words of "am, big" becomes "I am big boy".
Note: something is happening to the text above, there's suppose to be a blank between "I" and "a".
Third: I don't understand what "phrase" is. How is it determined and why does it need to be there?
Main.java
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
// write your code here
// TODO:csd - Instantiate a new Prompter object and prompt for the story template
Prompter prompter = new Prompter();
System.out.println("Write out a sentence in this manner __noun__adjective__, etc.");
String story = prompter.promptForStory();
Template tmpl = new Template(story);
// TODO:csd - Use the prompter object to have it do the prompting, censoring and outputting. Call Prompter.run
prompter.run(tmpl);
//List<String> fakeResults = Arrays.asList("friend", "talented", "java programmer", "high five");
// TODO:csd - This should really happen in the Prompter.run method, let's get these implemetation details out of the main method
}
}
Prompter.java
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
// TODO:csd - Print out the results that were gathered here by rendering the template
System.out.printf("Your TreeStory:%n%n%s", tmpl.render(results));
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException {
// TODO:csd - Prompt the user for the response to the phrase, make sure the word is censored, loop until you get a good response.
System.out.printf("Please enter a few words: %s", phrase);
String userWords = mReader.readLine();
while (mCensoredWords.contains(userWords.toLowerCase().trim())) {
System.out.printf("This language is not accepted, please don't say %s", userWords);
userWords = mReader.readLine();
}
return userWords;
}
public String promptForStory(){
System.out.printf("Enter a story using words separated with __: ");
String line = null;
try {
line = mReader.readLine();
} catch (IOException e) {
e.printStackTrace();
}
return line;
}
}

Kyle Baker
1,884 PointsI do understand how this is supposed to work better now, thank you, but it doesn't seem to be working as it should. In regards to my second question here is the result after running it:
Enter a story using words separated with __: I__a__ballerina.
Please enter a few words: aam beautiful
Your TreeStory:
Iam beautifulballerina.
Process finished with exit code 0
I put in "%s", phrase in the code after "Please enter a few words" and it inserted the middle word (a) of the template sentence. Also the final result cuts out "__ a __" of the template sentence and replaces it with the two entered words.
Thanks for your help!
1 Answer
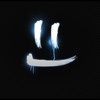
Grigorij Schleifer
10,365 PointsHi Kyle,
I think your problem is the understanding of the placeholders. For this challenge the placeholder is "name". The name inside of the "name" will be replaced and not __ .
If you look at the Template() method that accepts the story as a String with the placeholders ....... The word inside __ ...__ will be replaced.
Try this sentence:
I __word__ a __word__ ballerina.
and donΒ΄t forget the spaces between
Tell me if my idea is not working !
Grigorij
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHi Kyle,
First: You forgot to name the package in the main method
Second: What do you mean "the middle of the story"? Can you paste the results of the code please
Third: "phrase" is the String of the for loop that takes the placeholders ( name) from the template of the story. So every placeholder will be stored inside this String phrase. After this the phrase is given as parameter into the promptForWord(phrase) method. There the placeholders will be displayed to the user and the user can input his word for the placeholder.
Does it make sense?
Grigorij