Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial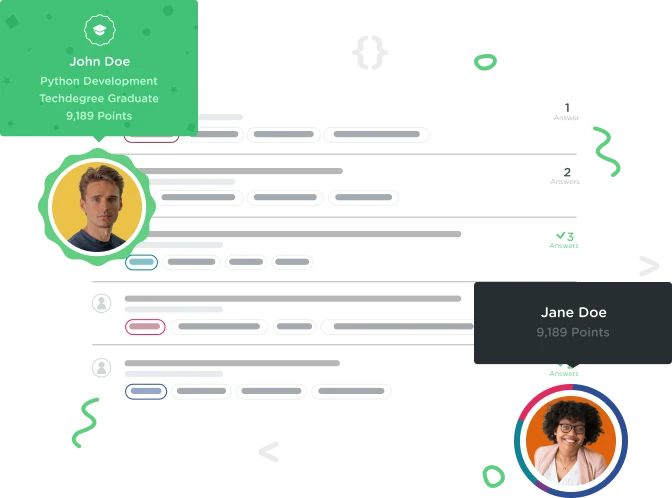
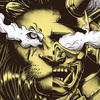
Joshua C
51,696 PointsAdd a static method named CalculateHypotenuse to the RightTriangle class
I've tried two different ways of calculating the hypotenuse and both ways, I get the error: "Bummer! Is your math correct?"
Am I calculating it wrong?
namespace Treehouse.CodeChallenges
{
class RightTriangle
{
public static double CalculateHypotenuse (double side1, double side2)
{
return System.Math.Pow(side1, 2) + System.Math.Pow(side2, 2);
//return (side1 * side1) + (side2 * side2);
}
}
}
4 Answers
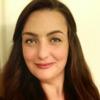
Jennifer Nordell
Treehouse TeacherYour code is great! Your math is a little off. Let's take a triangle with the sides 3, 4, 5. The formula is a^2 + b^2 = c^2. So 9 + 16 = 25. So if we want to solve for the hypotenuse we take the squares of both sides and add them, which you did perfectly. But then we take the square root of the result.
namespace Treehouse.CodeChallenges
{
class RightTriangle
{
public static double CalculateHypotenuse (double side1, double side2)
{
return System.Math.Sqrt(System.Math.Pow(side1, 2) + System.Math.Pow(side2, 2));
//return (side1 * side1) + (side2 * side2);
}
}
}
You are just missing one tiny math function. Hope this helps!
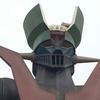
Alexandre C.
1,245 PointsCan somebody help me with my code? I read the answer already, but I didn't just want to copy-paste. Would my code ever work as it's been written (with some kind of get modification?) Thanks!
namespace Treehouse.CodeChallenges
{
class RightTriangle
{
private static double CalculateHypotenuse
{
double a = x;
Console.WriteLine("Insert the length of a:");
x = Console.Read();
double b = y;
Console.WriteLine("Insert the length of b:");
y = Console.Read();
return System.Math.Sqrt(System.Math.Pow(x*x) + System.Math.Pow(y*y);
}
}
}
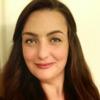
Jennifer Nordell
Treehouse TeacherYes, and no. First you would have to redo how you're getting and assigning the input from the user. But furthermore, it would only work if the user put in 2 for both sides. The reason being is that you have x to the power of x. This means if they enter 2 for x it would be 2 the the power of 2 (which is valid for the Pythagorean theorem). However, if the user put in 5 for x it would be 5 to the power of 5 when what you want is 5 to the power of 2. This would result in you having a value of 3125 where you should really only have a value of 25. That's a large difference. hope this helps!
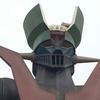
Alexandre C.
1,245 PointsThank you very much for your help, now I get it!

Matt Fredericks
8,367 PointsIf the point is to understand the concept and allow users to test their understanding, why turn this exercise into a math problem? Ugh....