Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial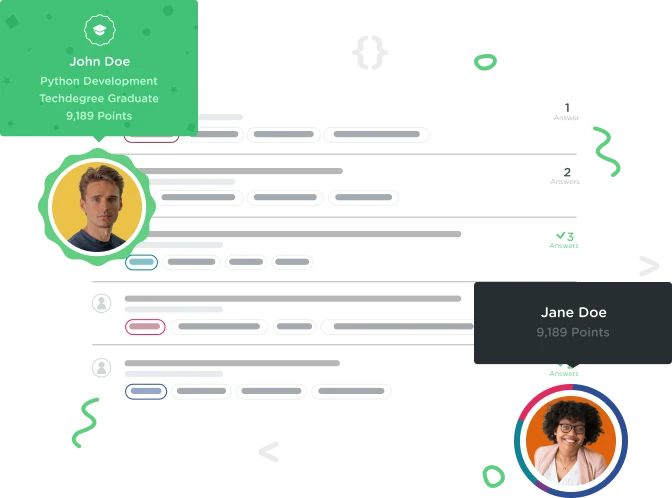

David Merk
9,863 PointsAdd an Equals method to the VocabularyWord class that returns true if the words of two VocabularyWord objects are the sa
Add an Equals method to the VocabularyWord class that returns true if the words of two VocabularyWord objects are the same.
I am lost. I really wish I weren't but somewhere I've deviated a lot from the path and now out of frustration I'm asking for guidance in this code challenge. I've tried a lot of things and received a plethora of compiler errors and wrong turn messages, however none of them have lead me to any conclusion besides frustration.
here is the code I have. I know it's pretty wrong, I've probably had other code which was less wrong, but I've watched the video pertaining to this a dozen times and I still don't get how to do what's being asked.
using System; namespace Treehouse.CodeChallenges { public class VocabularyWord { public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(object word)
{
if (Word.Equals(word))
{
return true;
}
else
{
return false;
}
}
}
}
5 Answers
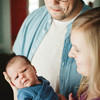
Luke Vaughan
15,258 PointsHi David,
Shannon's Answer is correct. I just thought you may want it all together in format.
I had a hard time with this one as well!
If you remember from the previous video he explains the reason that we cannot only use the == sign. Therefore we must use the Equals Method.
First you need to create the method make sure it is public do not forget override and it is a bool. I used obj as the name but you can use whatever you want. I figure obj is what the instructor used so it is easiest for me to use.
Then you need to write an if else. You want to make sure that the obj is equal the vocabulary word then you need to return it false. I have provided a link to give some insight on the "!" operator. https://msdn.microsoft.com/en-us/library/f2kd6eb2.aspx.
Then you need to set another var (you can name this what you want but I chose that because this is what the instructor chose). So this Var is going to = the obj as VocabularyWord;
Then you want to return this.Word and have it Equals(that.Word);
Im not sure if this is really all that helpful but this is what I try to do when I think through the problem. Take it for what you want. My explanation may not be 100% correct but it gets you thinking about how to read the code you are writing. And hopefully it will make you understand it as you write it.
public override bool Equals(object obj)
{
if(!(obj is VocabularyWord))
{
return false;
}
var that = obj as VocabularyWord;
return this.Word.Equals(that.Word);
}
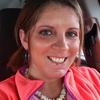
Shannon Beach
32,353 PointsTry this
public override bool Equals(object obj) { if(!(obj is VocabularyWord)) { return false; }
var that = obj as VocabularyWord;
return this.Word.Equals(that.Word);
}

Michael Kroon
16,255 PointsMy answer differs just a little from Luke's and Shannon's. I think Jeremy says that the equality operand "==" does work on numbers and strings because it checks the actual value of the variable. So, I was able to solve the challenge with this:
public override bool Equals(object obj)
{
if(!(obj is VocabularyWord))
{
return false;
}
else
{
VocabularyWord that = obj as VocabularyWord;
return this.Word == that.Word;
}
}
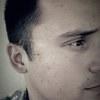
Brendon Brooks
8,802 Points@MichaelKroon
Why wouldn't this particular answer would not work?
public override bool Equals(object obj)
{
if(!(obj is VocabularyWord))
{
return true;
}
}
else
{
return false;
}

Michael Kroon
16,255 PointsBrendon,
I noticed three things with your code:
1) This part of the 'if statement' is a test to make sure that the object being passed in is at least a comparable object. Otherwise, why even try comparing the two, right? If it fails to be a comparable object, then you want it to return false.
if(!(obj is VocabularyWord))
{
return true;
}}
2) It looks like there's a double closing bracket between returning true and your 'else statement'. And it looks like there's a missing closing bracket to close the method? This would keep your code from compiling.
return true;
}}
else
{
3) The code needs to return true or false IF the Vocabulary.Word objects are the same. So there's need to be a comparison of the objects, not just returning a Boolean value.
else
{
return false;
}
Hope this helps! Let me know if this doesn't work. I'm still learning, too! :)
Michael

Joel Lundqvist
12,640 Pointscan someone link the full thing i can't get it to work
Adrian Torrente Tenreiro
12,540 PointsAdrian Torrente Tenreiro
12,540 PointsThis is causing me a massive headache... Why would you want to create a new var inside the override method if you want to compare a existing var(Word) with the object you passing to Override Equals method?
public override bool Equals(object word) { if(this.Equals(Word)) { return true; } return false; }
A native error showing up on the editor could someone please explain this to me? still done get it.