Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial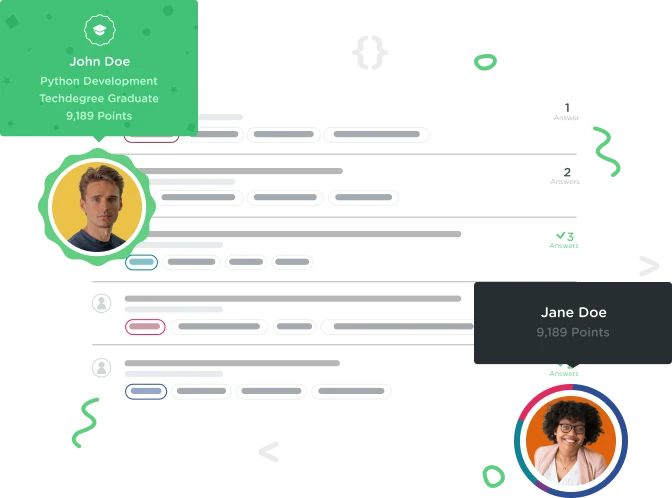

yahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsAdded a remove_item function to the code, any suggestions on what to add as well?
shopping_list = []
def add_item(item):
shopping_list.append(item)
print(f"your list has {len(shopping_list)} items in it")
return
def show_help():
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to display your full shopping list
Enter 'REMOVE' then enter the item you want removed
""")
def show_list():
print("here's your list: ")
for item in shopping_list:
print(item)
def remove_item(item):
remove_item = shopping_list.remove(item)
return
show_help()
while True:
new_item = input("> ")
if new_item.upper() == 'DONE':
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'REMOVE':
new_item = input('Enter item to be removed: ')
remove_item(new_item)
print(f"{new_item} has been removed, your list has {len(shopping_list)} items in it")
continue
elif new_item.upper() == 'SHOW':
show_list()
continue
add_item(new_item)
show_list()
3 Answers
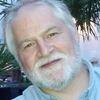
Jeff Muday
Treehouse Moderator 28,725 PointsHere is a simple realization of string list reading and writing. You will have to figure out what functions you need to copy into your program and where to use them.
Here is a "snapshot" of the workspace--
# a simple list plain-text read and write functions
def write_list_to_disk(mylist, filename='mylist.txt'):
# writes a string list to a file, default filename is 'mylist.txt'
with open(filename, 'w') as outfile:
# this writes each element on a new line
outfile.write('\n'.join(mylist))
def read_list_from_file(filename='mylist.txt'):
# function reads a string list from a file, each item is
# on a new line. And finally returns a NEW list
with open(filename, 'r') as infile:
data = infile.read()
mylist = data.split('\n')
return mylist
# time to test if it works--
# start with a shopping_list
shopping_list = ['milk','bread','eggs','carrots']
print('--write list to file--')
print(shopping_list)
# here is where we actually write the list to a file in the current
# directory called 'shopping_list.txt'
write_list_to_disk(shopping_list, 'shopping_list.txt')
# now, read it back to test it out
saved_list = read_list_from_file('shopping_list.txt')
print('--read list from file--')
print(saved_list)
Output looks like this:
treehouse:~/workspace$ python list_helper.py
--write list to file--
['milk', 'bread', 'eggs', 'carrots']
--read list from file--
['milk', 'bread', 'eggs', 'carrots']
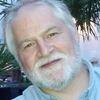
Jeff Muday
Treehouse Moderator 28,725 PointsThis is a nice approachable portfolio program. Keep up the good work!
It could be easily adapted to a TODO list app--
A couple of nice additions might be a LOAD and SAVE commands.

yahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsThank you Jeff! :) I will return to this code once I know how to add these commands :)
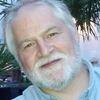
Jeff Muday
Treehouse Moderator 28,725 PointsIf you need any hints on how to implement, let me know.

yahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsThat would be awesome!, I still don't know how to import and export data into the code, I am sure it is covered later in the techdegree. But I'm sure learning how to do it now will be a great boost!
yahyaalshwaily2
Python Development Techdegree Graduate 20,049 Pointsyahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsThis is Awesome! Thank you Jeff!