Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial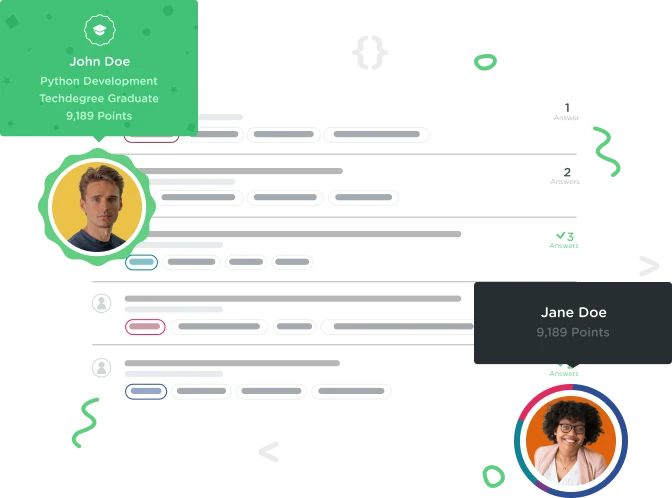
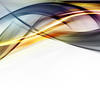
Scott Tucker
3,765 PointsASP .NET Comic Book Gallery project help!
This project is based off of the "Adding a Series List and Detail View" walkthrough that James Churchill created. You can find it here: https://github.com/treehouse-projects/aspnet-comic-book-gallery/tree/master/walkthroughs/add-series-list-and-detail-views.
The problem I am having is on the second to last step. It's the one where we create the Series Detail view. Below is the code I have for the Series Detail View. I have followed the walkthrough perfectly so I am confused on why I am having a problem.
@model ComicBookGallery.Models.Series
@{
ViewBag.Title = Model.Title;
ViewBag.ShowBackBar = true;
}
<h2>@ViewBag.Title</h2>
<div class="well">
<h5>Description</h5>
<div>
<p>@Html.Raw(Model.DescriptionHtml)</p>
</div>
</div>
<h4>Comic Books Available in This Series</h4>
@Html.Partial("_ComicBooksList", Model.Issues);
Whenever I run the project the, I can go to each comic book's detail page and view the list of comic books on the ComicBook Index page. I can even view the list of series. But when I click on one of the series, an exception comes up with this error "The model item passed into the dictionary is of type 'ComicBookGallery.Models.Series', but this dictionary requires a model item of type 'ComicBookGallery.Models.ComicBook[]'."
Through debugging I found that the Series.Issue property is null but I can't figure out why. Here is the GetSeriesDetail method which I think might be where the issue is.
public Series GetSeriesDetail(int id)
{
Series seriesToReturn = null;
foreach (var series in Data.Series)
{
if (series.Id == id)
{
seriesToReturn = series;
break;
}
if (seriesToReturn != null)
{
var comicBooks = new ComicBook[0];
foreach (var comicBook in Data.ComicBooks)
{
if (comicBook.Series != null && comicBook.Series.Id == id)
{
Array.Resize(ref comicBooks, comicBooks.Length + 1);
comicBooks[comicBooks.Length - 1] = comicBook;
}
}
seriesToReturn.Issues = comicBooks;
}
}
return seriesToReturn;
}
For more of the code, here is my GitHub repository for this project: https://github.com/ScottyT/comic-book-gallery/tree/master/src/ComicBookGallery.
Any help would be appreciated!
5 Answers

James Churchill
Treehouse TeacherScott,
Sorry to hear about your frustration with this exercise. I've updated the instructions for adding the SeriesRepository
class to include what the completed code should look like.
Check it out and let me know if you run into any further issues.
Thanks, James
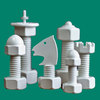
Steven Parker
241,985 PointsThe code above looks good. But I did the same course, so I compared your sources to mine and found one significant difference in Views/Shared/_Layout.cshtml where you have this line:
@Html.ActionLink("Return to List", "Index", null, new { @class = "btn btn-default" })
But when the Series was added, this was given an explicit reference to the ComicBooks controller, like this:
@Html.ActionLink("Return to List", "Index", "ComicBooks", null, new { @class = "btn btn-default" })
I would only expect that to go to the wrong location when the "Return" button was used, not do what you describe, so I'm still not sure what's causing your issue.
Is it possible your sources and objects are out of sync? Have you tried a "rebuild all"?
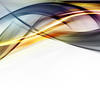
Scott Tucker
3,765 PointsI have rebuilt the ComicBookGallery solution and nothing changes. It still gives an exception that says: "The model item passed into the dictionary is of type 'ComicBookGallery.Models.Series', but this dictionary requires a model item of type 'ComicBookGallery.Models.ComicBook[]'." The property Issue is of type ComicBook[] but the model definition for the series detail view is of type Series., at least I think that's how you would describe it. I have tried changing the definition to ComicBookGallery.Models.ComicBook[] but that doesn't fix it.
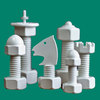
Steven Parker
241,985 PointsIf you're debugging, doesn't it show you exactly where this is happening? And before you make the click that causes the error, what does your browser show as the address where the link will go?
And are you sure your GitHub code is in sync with what you are building?
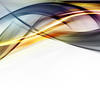
Scott Tucker
3,765 PointsWhen I am on the page that lists all the series, hovering over the first link, which is The Amazing Spider-Man, the browser shows the address as "/Series/Detail/1".
The below code works when the partial isn't referenced.
@model ComicBookGallery.Models.Series
@{
ViewBag.Title = Model.Title;
ViewBag.ShowBackBar = true;
}
<h2>@ViewBag.Title</h2>
<div class="well">
<h5>Description</h5>
<div>
<p>@Html.Raw(Model.DescriptionHtml)</p>
</div>
</div>
<h4>Comic Books Available in This Series</h4>
@Html.Partial("_ComicBooksList", Model.Issues);
If the last line of code is left out, then I can get to the Detail page for both "The Amazing Spider-Man" and "Bone", but the pages won't show the comic books available in the series. The source for where the error is happening is is the Series/Detail.cshtml file and it is with the @Html.Partial(). I hope I am making sense.
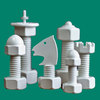
Steven Parker
241,985 PointsYou're making sense, but the problem isn't. Clearly, the Shared/_ComicBoosList.cshtml requres a type ...ComicBook[]. But how is it being passed a ...Series? In Series/Detail.cshtml your call to Html.Partial passes Model.Issues as a 2nd argument, which is a ...ComicBook[] type.
It's behaving like you had Model in that 2nd argument instead of Model.Issues. That's why I was wondering if perhaps your source differs from the one above or in GitHub.
Otherwise, I'm baffled.
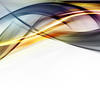
Scott Tucker
3,765 PointsI just checked and all of files are synced to GitHub. I have double and triple the walkthrough to see if I was missing something and I wasn't. I'm baffled too because I would think other people are having the same problem with this walkthrough but it seems like I am the only one.
public Series GetSeriesDetail(int id)
{
Series seriesToReturn = null;
foreach (var series in Data.Series)
{
if (series.Id == id)
{
seriesToReturn = series;
break;
}
if (seriesToReturn != null)
{
var comicBooks = new ComicBook[0];
foreach (var comicBook in Data.ComicBooks)
{
if (comicBook.Series != null && comicBook.Series.Id == id)
{
Array.Resize(ref comicBooks, comicBooks.Length + 1);
comicBooks[comicBooks.Length - 1] = comicBook;
}
}
seriesToReturn.Issues = comicBooks;
}
}
return seriesToReturn;
}
As the instructor said, we're suppose to loop through the available comic books and check to see if the comic book has a series and its Id property equals our id argument value. When we find a matching comic book, we'll add it to a local array variable. When I was debugging and I clicked on "Bone", in the Autos window in Visual Stuido the Issues property had a value of null. Here is my code for the Series model:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace ComicBookGallery.Models
{
public class Series
{
public int Id { get; set; }
public string Title { get; set; }
public string DescriptionHtml { get; set; }
public ComicBook[] Issues { get; set; }
}
}
And the ComicBook model:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace ComicBookGallery.Models
{
public class ComicBook
{
public int Id { get; set; }
public Series Series { get; set; }
public int IssueNumber { get; set; }
public string DescriptionHtml { get; set; }
public Artist[] Artists { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
var series = Series;
if (series != null)
{
return series.Title + " #" + IssueNumber;
}
else
{
return null;
}
}
}
// series-title-issuenumber.jpg
public string CoverImageFileName
{
get
{
var series = Series;
if (series != null)
{
return series.Title.Replace(" ", "-")
.ToLower() + "-" + IssueNumber + ".jpg";
}
else
{
return null;
}
}
}
}
}

James Churchill
Treehouse TeacherScott,
You're spot on about the Series.Issue
property being null, when it shouldn't be. The error message that the debugger is displaying, to be honest, is really just confusing the issue (unfortunately that sometimes just happens).
Have you tried setting a breakpoint just inside of your SeriesRepository.GetSeriesDetail
method so that you can step through your code line by line? As soon as I did that, I noticed a problem with how that method was behaving.
Give it a go and let me know what you discover. Don't struggle with it too much; I can always give you another hint :)
Thanks, James
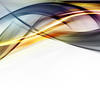
Scott Tucker
3,765 PointsAlright, I set a breakpoint just inside the GetSeriesDetail method. It looks like Data.Series is returning the two series and when I clicked on "Bone" the id displays as 2, which it should. I noticed that seriesToReturn is always null. I have tried to change null to new Series(), new ComicBook() and still seriesToReturn is null.
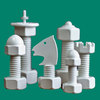
Steven Parker
241,985 PointsAre you sure seriesToReturn is null? That gets set when the ID matches.
Or is it seriesToReturn.Issues that is null?
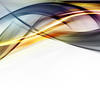
Scott Tucker
3,765 PointsYou are correct Steven. It is the seriesToReturn.Issues that is null. I clicked on Bone while I was debugging and that has an id of 2 so I had to step through the code a second time to see the seriesToReturn.Issues.
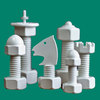
Steven Parker
241,985 PointsOh dang, I got totally distracted by the error message and forgot about the null value. But I feel totally vindicated now about questioning if the sources were in sync!
So, in the code you included above for GetSeriesDetail you have this line:
var comicBooks = new ComicBook[0];
But in your GitHub code you have it like this:
var comicBooks = new ComicBook[1];
Now guess what the first item in that array is going to contain?
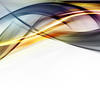
Scott Tucker
3,765 PointsIn a previous version I had a 1 in the brackets. Just this afternoon I changed it back to 0 but even then, the problem still remains.
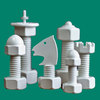
Steven Parker
241,985 PointsOk, so just because my guess about the sources out of sync was right doesn't mean that's the only issue.
So now try James' suggestion of stepping through and see what it does.
Hint: Pay close attention to what happens (or doesn't happen) in the loop.
Extra hint: The problem is the result of a closing brace ("}
") in the wrong place.
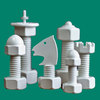
Steven Parker
241,985 PointsWhew! All that downloading and comparing was a lot of work ... I hope it helped a little bit.
I'll unfollow now that you've chosen the best answer.
Scott Tucker
3,765 PointsScott Tucker
3,765 PointsThe updated walkthrough really helped! The answer was starring me right in my face. I didn't think about taking the if(seriesToReturn != null) statement outside of the foreach loop because I thought seriesToReturn was already assigned the current series. Thank you so much! :)
Near the beginning of this step where you compared the GetComicBook method with the GetSeriesDetail method, the code for the GetComicBook method has Data.ComicBooksData as the loop source. It should be Data.ComicBooks like it originally was. Other than that, I don't have any further issues. Thanks!
James Churchill
Treehouse TeacherJames Churchill
Treehouse TeacherScott,
Thanks for letting me know about the misnamed property! I just fixed that error in the walkthrough :)
Thanks, James