Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial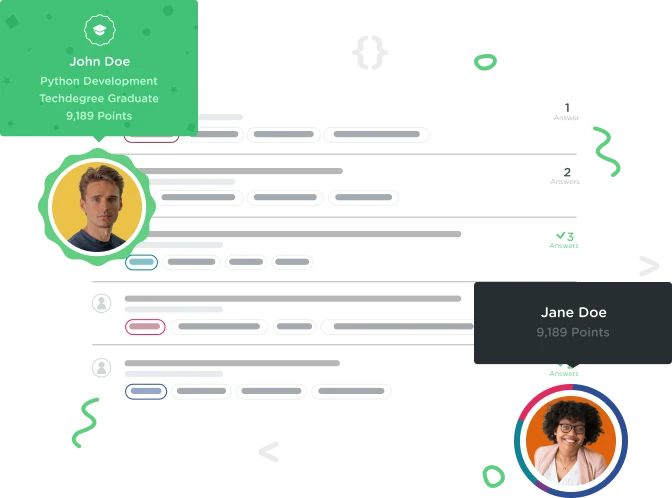

Anthony Costanza
2,123 Pointsassertion error
What am I doing wrong here?
"""
This is importing a function named `tweet` from a file
that we unfortunately don't have access to change.
You use it like so:
>>> tweet("Hello this is my tweet")
If the function cannot connect to Twitter,
the function will raise a `CommunicationError`
If the message is too long,
the function will raise a `MessageTooLongError`
"""
from twitter import (
tweet,
MessageTooLongError,
CommunicationError,
)
message = input("What would you like to tweet? ")
# Your code here
tweet(message)
try:
print(message)
except CommunicationError:
print("Communication Error")
except MessageTooLongError:
print("Your message is too long")
1 Answer
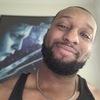
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsHi Anthony Costanza,
Your issue with the second challenge has to do with your try...except block. There is no CommunicationError because you’re trying to invoke the print function and not the tweet function.
Well, technically your tweet function isn’t placed within a try...except block, so when there is a CommunicationError before the try...except block where you attempt the print(message) function, there is no exception to handle it. So that’s technically why you’re getting the assertion error.
But the bigger issue is that in your try block you should be calling tweet(message) not print(message). So move your tweet(message) into your try block, and then you can delete the print(message) statement altogether. Do that and you’ll be on your way to passing the second challenge (although you’ll still need to change the message you’re printing to the console to the one the challenge asks you to use).
With the third challenge, in addition to changing the print message to the correct message, you'll need to include the message returned from the exception. You'll do that using the following syntax except MessageTooLongError as err:
, with err simply being a variable to hold the exception. You'll then plug it into the print message.
Anthony Costanza
2,123 PointsAnthony Costanza
2,123 PointsOk - I will try that - thank you!
Anthony Costanza
2,123 PointsAnthony Costanza
2,123 PointsHi - I tried that and I'm still getting the error
from twitter import ( tweet, MessageTooLongError, CommunicationError, )
message = input("What would you like to tweet? ")
Your code here
try: tweet(message)
except CommunicationError: print("Communication Error")
except MessageTooLongError: print("An error occurred attempting to connect to Twitter. Please try again!")
Anthony Costanza
2,123 PointsAnthony Costanza
2,123 PointsOk - I got it - I didn't realize you have to print to the console exactly what the challenge is asking - thank you!!