Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial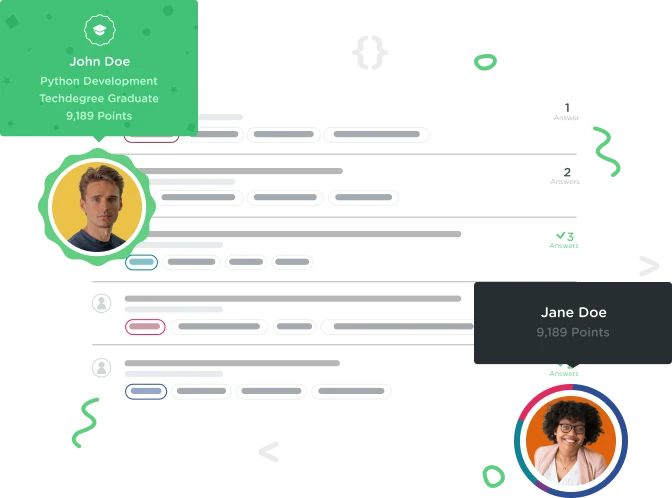
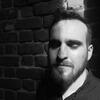
matthew mahoney
Python Development Techdegree Student 2,536 PointsBudget Calculator. Not running/Reformatting suggestions
def budget(monthly_income):
max_mortgage = int(monthly_income) * .25
savings_retirement = int(monthly_income) * .10
food = int(monthly_income) * .15
giving = int(monthly_income) * .10
utilities = int(monthly_income) * .5
transportation = int(monthly_income) * .10
health = int(monthly_income) * .5
insurance = int(monthly_income) * .10
recreation = int(monthly_income) * .5
misc = int(monthly_income) * .5
print(f"Your maximum monthly mortgage or rent payment should not exceed ${max_mortgage}.")
print(f"You should try to save ${savings_retirement} a month.")
print(f"Try to keep your food budget around ${food} a month or ${food / 4} a week.")
print(f"Giving is an important aspect of growing wealth. We reccomend that you give around ${giving} a month.")
print(f"Try to keep your monthly utilities costs around ${utilities}.")
print(f"Costs for transportation, gas and upkeep of your car should not exceed ${transportation} monthly")
print(f"Health costs, such as perscriptions and vitamins should be kept at ${health} a month.")
print(f"Insurances expeanses, like car and health should be capped at ${insurance}.")
print(f"Fun money would be good kept at ${recreation}.")
print(f"Misc expenses, things unseen but planned for, we should set aside ${misc} a month.")
monthly_income = input("Okay, what is your total monthly, after-tax, take-home pay? Including your spouse's income. ")
1 Answer
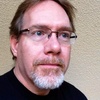
Chris Freeman
Treehouse Moderator 68,426 PointsHey matthew mahoney, it appears you arenβt calling the function budget
with the input value.
As for coding style, there are many redundant calls to int()
. Perhaps convert before calling the function and pass the int
, or create a local int
inside the function and reuse it.
The code might be more readable to combine all the print
statements into a single statement. multiline f-strings are possible as long as there is a wrapping paren. Luckily, the print
function call provides them for us.
print(f"Your maximum monthly mortgage or rent payment should not exceed ${max_mortgage}.\n",
f"You should try to save ${savings_retirement} a month.\n",
f"Try to keep your food budget around ${food} a month or ${food / 4} a week.")
Notice the added \n to cause a line break as needed. You may also want to wrap lines at the 80 character mark
Post back if you need more help. Good luck!!!
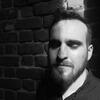
matthew mahoney
Python Development Techdegree Student 2,536 PointsAmazing! Thanks so much! Also, I forgot a decimel point on some of these! Who wants to spend 1900 on utilities lol
matthew mahoney
Python Development Techdegree Student 2,536 Pointsmatthew mahoney
Python Development Techdegree Student 2,536 PointsBased on the recommended amounts here: https://struggle.co/dave-ramsey-budget-percentages/