Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial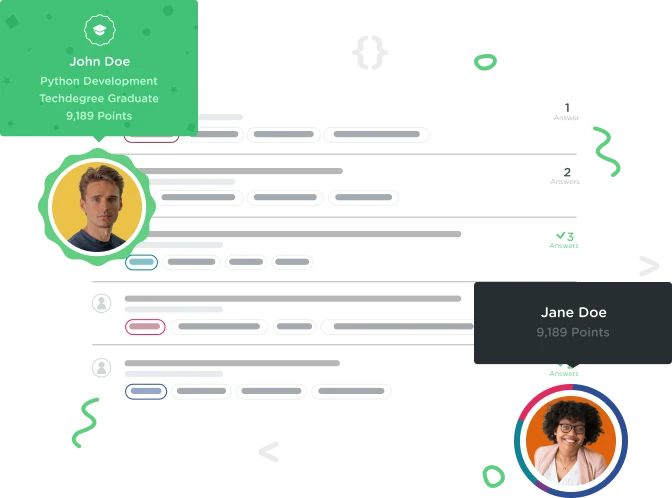

Dov Brodkin
2,695 PointsC# null value
Here is my code using System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { restart: Console.Write("Enter the number of times to print \"Yay!\": "); string userInput = Console.ReadLine();
try{
int loopYay = int.Parse(userInput);
if (loopYay<0)
{
Console.WriteLine("You must enter a positive number.");
goto restart;
}
while(loopYay>0) {
Console.WriteLine("Yay!");
loopYay--;
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number");
}
}
}
}
And the program returns
System.ArgumentNullException: Value cannot be null. Parameter name: String at System.Number.StringToNumber (System.String str, NumberStyles options, System.NumberBuffer& number, System.Globalization.NumberFormatInfo info, Boolean parseDecimal) [0x00054] in /builddir/build/BUILD/mono-4.2.3/external/referencesource/mscorlib/system/number.cs:1080 at System.Number.ParseInt32 (System.String s, NumberStyles style, System.Globalization.NumberFormatInfo info) [0x00014] in /builddir/build/BUILD/mono-4.2.3/external/referencesource/mscorlib/system/number.cs:751 at System.Int32.Parse (System.String s) [0x00000] in /builddir/build/BUILD/mono-4.2.3/external/referencesource/mscorlib/system/int32.cs:140 at Treehouse.CodeChallenges.Program.Main () <0x415eac00 + 0x00046> in :0 at MonoTester.Run () [0x002b3] in MonoTester.cs:169 at MonoTester.Main (System.String[] args) [0x00013] in MonoTester.cs:28
Why is that? What did I do wrong?
1 Answer
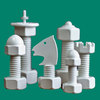
Steven Parker
231,236 Points
ReadLine() will return null if it is unable to get any input.
Normally it would wait for you to enter something. Did you type an End_of_File character at the prompt? Or was the input stream closed or redirected? Or maybe the input stream got set to a non-blocking mode?
To avoid the error, you could move the ReadLine into the try block and add another catch for ArgumentNullException, but then that won't help determine what caused it to begin with.
Dov Brodkin
2,695 PointsDov Brodkin
2,695 PointsThis is a code challenge for C# basics (last one). I did not type an End_of_File character at the prompt. I'm not sure about the input stream etc. (don't even know what it is). Thank you for your help (I rewrote the whole thing in the try block with slightly different code, and everything worked.