Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial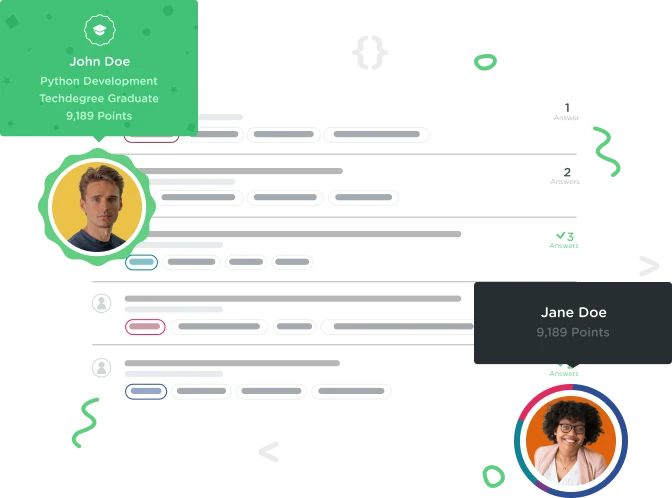

mcutz
8,063 PointsC# Objects Catching Exceptions - What's wrong with this code?
The questions reads:
In the Program.cs file, write code to check if the value integer is less than 0 or greater than 20. Throw System.Exception if the value is outside of the range.
URL: Challenge
My Answer:
int value = int.Parse(Console.ReadLine());
try
{
if (value < 0 || value > 20)
{
throw new System.Exception();
}
Console.WriteLine(string.Format("You entered {0}", value));
}
catch(Exception)
{
Console.WriteLine("Not within bounds!");
}
Result:
Bummer! Did you throw an exception when the value is less than 0 or greater than 20?
What am I missing here?
Thanks
9 Answers

anil rahman
7,786 PointsThere you go
int value = int.Parse(Console.ReadLine());
if(value < 0 || value > 20)
{
throw new System.Exception();
}
Console.WriteLine(string.Format("You entered {0}",value));

anil rahman
7,786 PointsWhen you are on the challenge itself, you click get help and ask your question straight from there and the challenge will get linked :)

mcutz
8,063 PointsSo I don't try to catch it? Ha was way over thinking it!
Thank you very much!

anil rahman
7,786 PointsTry removing the console.writeline under the if.
I can't attempt the code as you didn't link the challenge.

mcutz
8,063 PointsNot sure if that was what you had in mind but added the URL of the challenge above the code.

mcutz
8,063 PointsAh OK, will remember that for next time, thanks. Don't want to make a duplicate post, so will the URL link I've added above the code help?

anil rahman
7,786 PointsYh thats fine

anil rahman
7,786 PointsYh the question would have mentioned it if needed :)
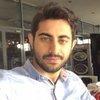
HIDAYATULLAH ARGHANDABI
21,058 Pointsint value = int.Parse(Console.ReadLine());
if(value < 0 || value > 20)
{
throw new System.Exception();
}
Console.WriteLine(string.Format("You entered {0}",value));