Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial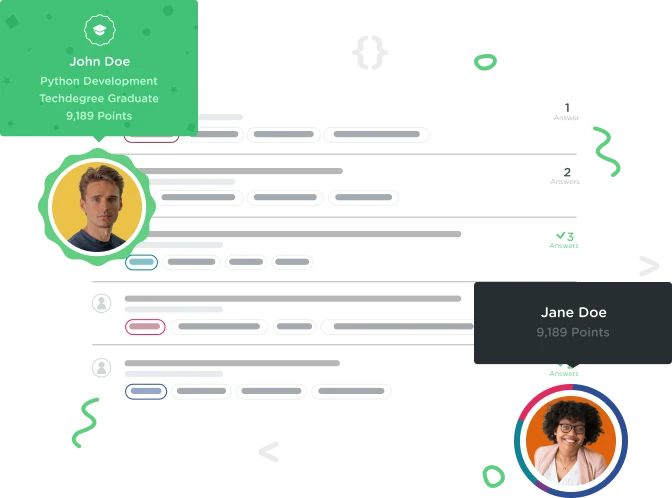
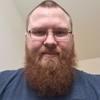
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsC# Speech.Recognition
I'm trying to make a simple virtual "butler" (like jarvis) for the purpose of learning new things about c#.
Two days ago I realized that I can't use System.Speech.Recognition due to I don't have an english version of windows. I made it work by using the Microsoft.Speech.Recognition instead.
My problem is that I'd love to make it convert speech-to-text -> store it in a variable -> then use it for something like "search for 'variable'".
I've been looking all over google, youtube, msdn, stackoverflow etc for two days now, and nothing seems to work :-/
I'm really sure that I should be using .AppendDictation() since I'm using microsoft.speech, but the example from msdn is not working in my case (I might be using it wrong)
GrammarBuilder startStop = new GrammarBuilder();
GrammarBuilder dictation = new GrammarBuilder();
dictation.AppendDictation();
startStop.Append(new SemanticResultKey("StartDictation", new SemanticResultValue("Start Dictation",true)));
startStop.Append(new SemanticResultKey("DictationInput", dictation));
startStop.Append(new SemanticResultKey("StopDictation", new SemanticResultValue("Stop Dictation", false)));
Grammar grammar=new Grammar(startStop);
grammar.Enabled=true;
grammar.Name=" Free-Text Dictation ";
_recognizer.LoadGrammar(grammar);
I hope there is someone in here that would take a minute to tell me if I'm on the right track, where to look or even give an example of to get it work.
1 Answer
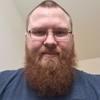
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsHere is my current code and it's working just fine - the computer can speak, recognize the words I've put into the grammar etc., but still can't figure out how to use that code pasted in the post above in my current code.
My current code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using Microsoft.Speech.Synthesis;
using Microsoft.Speech.Recognition;
namespace Meeko
{
public partial class Form1 : Form
{
SpeechSynthesizer meeko = new SpeechSynthesizer();
SpeechRecognitionEngine sr = new SpeechRecognitionEngine();
private string Greet { get; set; }
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
meeko.SetOutputToDefaultAudioDevice();
meeko.SelectVoice("Microsoft Server Speech Text to Speech Voice (en-GB, Hazel)");
Choices inputs = new Choices();
inputs.Add(new string[] { "hello", "goodbye" ,"my name is" });
GrammarBuilder gb = new GrammarBuilder();
gb.Append(inputs);
Grammar g = new Grammar(gb);
sr.SetInputToDefaultAudioDevice();
sr.LoadGrammarAsync(g);
sr.SpeechRecognized += sr_SpeechRecognized;
sr.RecognizeAsync(RecognizeMode.Multiple);
}
void sr_SpeechRecognized(object sender, SpeechRecognizedEventArgs e)
{
MessageBox.Show("Speech recognized: " + e.Result.Text);
if (e.Result.Text == "my name is")
{
// store the users name in a variable
}
}