Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial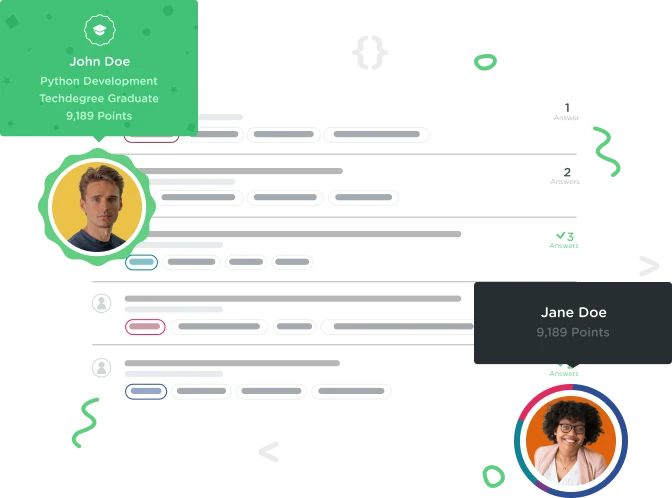
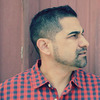
Juan Gallardo
1,567 PointsCall the print function on the console object and make it print out "<YOUR NAME> can code in Java!"
Stuck here. This is what I attempted
// I have setup a java.io.Console object for you named console
String firstName = "Juan";
console.printf("%s", firstName + "can code in Java");
6 Answers
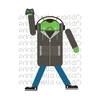
Stephen Bone
12,359 PointsHi Juan
Ok so you've almost got your print statement correct you just need to change the order a little bit.
So you have to include the %s placeholder as part of string and then pass in the value you would like to replace it seperated by a comma afterwards.
console.printf("%s can code in Java!", firstName);
Hope it helps!
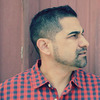
Juan Gallardo
1,567 PointsOk I see where I made the mistake. I got it to work with this
console.printf("%s can code in Java!\n", firstName);
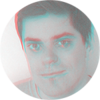
Marcos Frutos
1,291 PointsI'm blocked here too.
String firstName = "Mark";
console.printf("%s is coding in Java!", firstName);
EDIT: Oh sorry about that, I had to write the exact words.

william parrish
13,774 Pointsconsole.printf("%s can code in Java!", firstName);
You were close, remember that its no problem to put the variable to be interpolated in, and continue with the regular string characters, without breaking out of the string. After you have closed the String "" you then put in the appropriate number of variables that need to be interpolated / inserted.
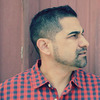
Juan Gallardo
1,567 Pointsso if it had multiple variables, would it be something like
console.printf("%s can code in Java!\n", firstName + lastName);
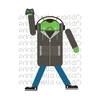
Stephen Bone
12,359 PointsIn this case it would be:
// pretending of course that there is another variable called language
console.printf("%s can code in %s!", firstName, language);
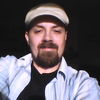
Robert Manolis
Treehouse Guest Teachershould it be var instead of string?
should it be console.printf? that last little f there might be messing you up.
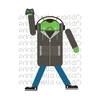
Stephen Bone
12,359 PointsHi Robert
Yes It is supposed to be String and console.printf,
I think you are probably thinking of Javascript which is quite different.
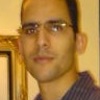
Samuel Mayol
4,786 PointsVery easy, look this
import java.io.Console;
public class PrintYourName {
public static void main(String[] args) {
Console console = System.console();
// Your code goes below here
String firstName = "Sam";
console.printf("Hello, my name is %s\n", firstName);
}
}
You have to import thee console input/output using: import java.io.Console; Then create a class and set a name for that class, I used: public class PrintYourName { }, do not forget the { } to create a class block of code. Inside of the { } curly braces goes the body of your class. The body of the class contain the main method: public static void main(String[] args) { }, the main method is run by the JVM, it is where your code start running. In the main method include the body block for this method.
console.printf("%s is learning how to write Java\n", firstName);
Create an instance of the Console imported like this: Console console = System.console(); Create a local variable type string: String firstName = "Sam"; This is just a single line comment, it is mandatory: // Your code goes below here We are using the object created after instantiate our Console class like this: console.printf("Hello, my name is %s\n", firstName);
console is an instance of Console and it has a method called 'printf' inside of this method we added an argument: "Hello, my name is %s\n", firstName
where "Hello, my name is %s\n" it is going to be display on the screen and the variable firstName also, however in this case the value of firstName variable will be displayed next to Hello, my name is, because we are using %s which is a format specifier for string values, then \n is a separator that perform a new line, after the " " double quotes goes a comma to separate the variable of the literal. The literal is again "Hello, my name is". The value of the variable is substituted for the %s. The result will be:
Hello, my name is Sam
To see how formatted strings works here I provide a link: [example link]http://www.homeandlearn.co.uk/java/java_formatted_strings.html
I hope to be clear in my example and you can understand my explanation, if you are not so familiar with the term that I used try to google it, because I do not want to do this comment too long.
s ss
115 Pointss ss
115 PointsString firstName = "Jay"; console.printf ("%s can code in Java!", firstName);