Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial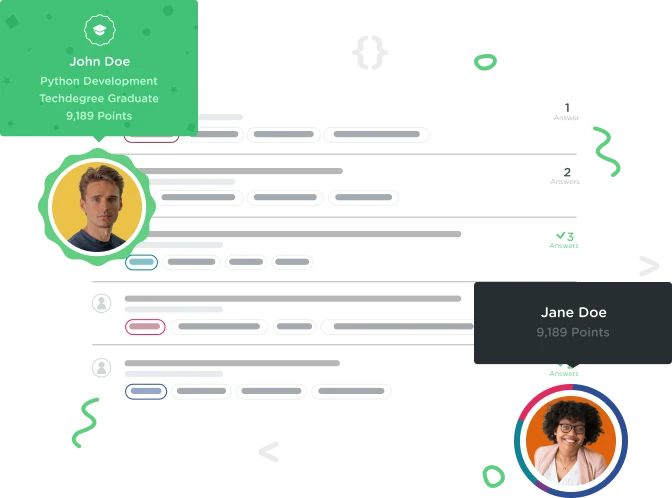

Josephine Hedman
6,462 PointsCan someone explain why the functions end up with the value 65 in the last example?
Hi, in the example of CPS (continuation-passing style), they give an example of functions that takes callbacks as arguments. Could someone be so kind and explain to my how the functions end up with the value 65 in the end? I've tried to go through the sequences but I'm not really getting it.
Thanks in advance!
2 Answers
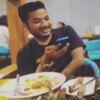
Ashish Mehra
2,411 Pointsfunction add(x, y, callback) {
// The x will be 5 and y will be 10 so sum will be 15
// The sum value will be forwarded to callback and in callback we're calling subtract
callback(x + y)
}
function subtract(x, y, callback) {
// The x will be 15 and y will be 2 so subtraction will be 13
// The subtracted value will be forwarded to callback and in callback we're calling mutiply
callback(x - y);
}
function multiply(x, y, callback) {
// The x will be 13 and y will be 5 so product will be 65
// The product value will be forwarded to callback and the n will be 65
callback(x * y);
}
function calculate(x, callback) {
// The x will be 5
//Value of 5 will be forwarded to callback & in callback we're calling add with 5
callback(x);
}
calculate(5, (n) => {
add(n, 10, (n) => {
subtract(n, 2, (n) => {
multiply(n, 5, (n) => {
console.log(n); // 65
});
});
});
});
Hope it helps. Let me know if you have still any doubts.
Thanks

Josephine Hedman
6,462 PointsThanks a lot! I get it now. Had some trouble understanding the order in which to read the callback function so it helped a lot by seeing it step by step :D
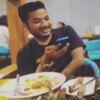
Ashish Mehra
2,411 Pointscheers :)