Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial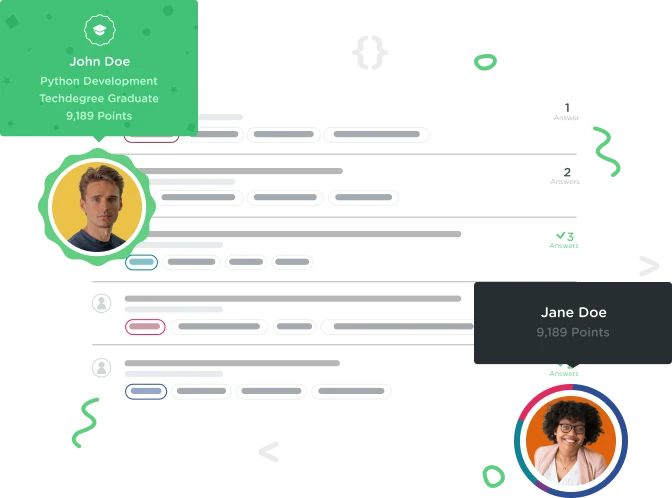

jose rodriguez
16,524 PointsCan someone Please Help!! I just do not see what I am doing wrong for this function!!
I placed this code in this way and thought that maybe it would work but its failing. I even added it to the end of the append line and that did not work. what other way should this be written?
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def add_item(self, item):
super().add_item(item)
self.slots.append(item)
self.slots.sort()
2 Answers
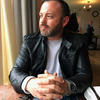
dmzstudio45
43,976 PointsHi Jose,
Since the add_item method is already defined in Inventory class, you only add new code to the add_item method in the SortedInventory class.
The line: super().add_item(item) added "self.slots.append(item)" to the method, so you didn't have to type that again.
So your SortedInventory class should look like:
class SortedInventory (Inventory) :
def add_item (self, item) :
super().add_item(item)
self.slots.sort()
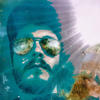
Casey Nord
12,141 PointsI ran into the exact same problem and my code looked identical to Jose's.
The second step in this Code Challenge lets you pass with this code:
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def add_item(self, item):
super().add_item(item)
self.slots.append(item)
However, on the third step it keeps giving you an "items don't appear to be sorted" message.
As David said in his post above, the line "self.slots.append(item)" doesn't need to be added to the SortedInventory class.
If you run this code in the shell you will find that every item added to the list is added twice.
>>> from inventory import SortedInventory
>>> bag = SortedInventory()
>>> bag.add_item(2)
>>> bag.add_item(5)
>>> bag.add_item(1)
>>> bag.add_item(12)
>>> bag.slots
[1, 1, 2, 2, 5, 5, 12, 12]
Using the code that David posted results in only one copy of each item being added, which is how it is intended to work, and will pass the challenge!
Hopefully this will help anyone who is having trouble understand how this is working!