Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial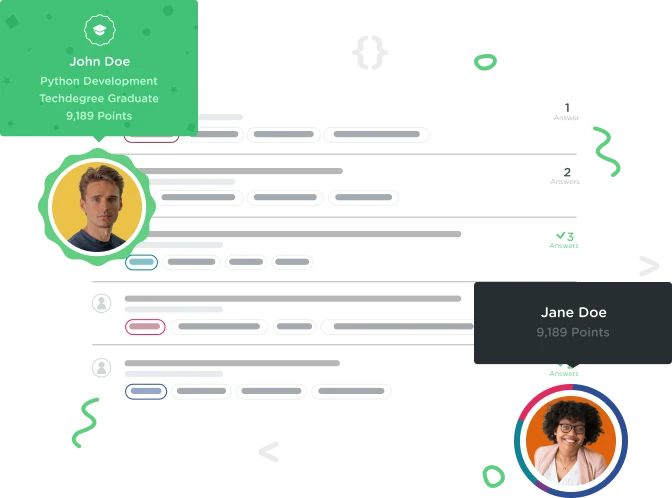

Dinesh kumar
2,777 Pointscan you help with this logic?
Create a new method named getCountOfLetter that returns an int, and requires a parameter of type char named letter. For this task, just make it return 0.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public int getCountOfLetter(char letter){
return(0);
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
8 Answers

markmneimneh
14,132 PointsHi
You probably means
return('O');
Hope this helps.
If this answers your question, please mark the question as answered.
Thanks

chase singhofen
3,811 Pointsthis worked for me
public int getCountOfLetter(char letter) { return 0; }
i 1st put public void and then replaced it with int good luck if u still need it

markmneimneh
14,132 PointsHi Marcia
I mis-read the zero as letter O
you are right, since the return val is int, then it is a zero.
Regards

Dinesh kumar
2,777 PointsThank you.

markmneimneh
14,132 PointsThere are many ways to do this. The basic approach is something like this (not tested)
public int getCountOfLetter(char letter){ int count = 0; for (int i=0; i < tiles.length(); i++) { if (tiles.charAt(i) == letter) { count++; } } return count; }
other more advanced approached is to use Lamda expression or may ve splitting the string. I believe the above should work for you.
if this answers your question, please mark the question as answered.

Mohit Ladia
4,140 Pointspublic int getCountOfLetter(char letter){ int count = 0; for (int i=0; i < tiles.length(); i++) { if (tiles.charAt(i) == letter) { count++; } } return count; } The above logic is not working , it is saying to use enhanced for each loop and use toCharArray() method. Please help me with this.

markmneimneh
14,132 Pointspublic int getCountOfLetter(char letter){
int count = 0;
for (int i=0; i < tiles.length(); i++) {
if (tiles.charAt(i) == letter) {
count++;
}
}
return count;
}
Try this for loop instead.
for (char c : tiles.toCharArray()) {
if (c == letter) {
++count;
}
}
return count;
I hope this wll point you in right direction

Mohit Ladia
4,140 PointsThanks
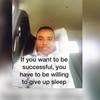
abdijabar sambul
1,063 Pointspublic int getCountOfLetter(char letter){ int count=0; for(char tile : tiles.toCharArray()){ if(tile==letter){ count++; } } return count; } }

Anthony Khoo
1,238 PointsHi, for the getCountOfLetter() method, in the char array for the if condition, I used the hasTile() method which we previously implement, which checks if the tiles have what we want.
Eg. of the hasTile() method. return tiles.indexOf(tile) != -1;
So now, for my GCOL() method, whereby in my if condition I used the following.
Eg. of GCOL method. public int getCountOfLetter(char letter) { int count = 0; for (char c : tiles.toCharArray()) { if (hasTile(letter)) { count++; } } return count; }
Am I doing something wrong with my syntax or logic? Thanks in advance.
Dinesh kumar
2,777 PointsDinesh kumar
2,777 Pointscan you help me with this,
Now in your new method, have it return a number representing the count of tiles that match the letter that was passed in to the method. Make sure to check Example.java for some example uses.
Marcia L.
4,686 PointsMarcia L.
4,686 PointsSince the return type is int, it doesn't seem like single quotes would be appropriate for the return statement. But I am not sure what Dinesh's question is. His method takes a char and returns the int zero, as per the instructions. But the instructions are ambiguous,,,do they want him to take a stab at the logic of the method, but in a way that causes it to (undesirably) always return zero, or do they just want him to put in that return statement as a placeholder?