Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial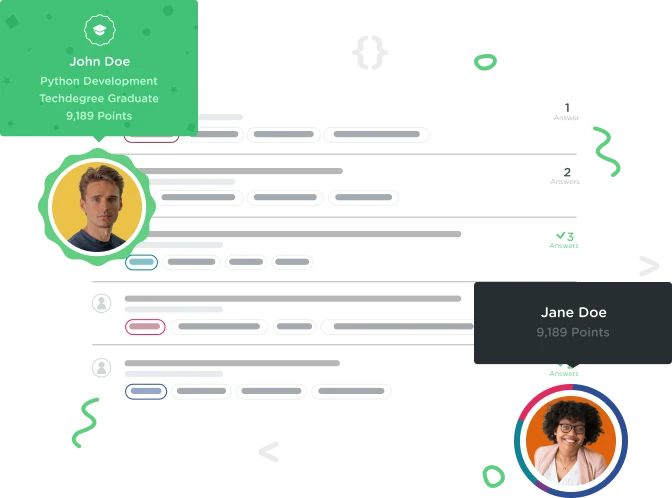

Harsh Khandelwal
5,539 PointsCannot understand behavior of this code.
For the below code
var Portland = {
bridges: 12,
airport: 1,
soccerTeams: 1,
logNumberOfBridges: function() {
console.log("There are " + this.bridges + " bridges in Portland!")
},
logTeams: function(){
console.log(this.logNumberOfBridges());
}
}
Portland.logTeams();
Why do I get undefined in the output?
Output:
There are 12 bridges in Portland!
undefined
3 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsThe developer tools in browsers like to be helpful, so they log the return value of a function/method in the console. When there is no return
statement in the function, the return value is undefined
, as is the case here. That's why the console shows undefined
.
Nothing is wrong with your code, it's just your browser trying to be helpful. You might notice that there's a little arrow in front of undefined
— this signifies that it's a return value.
If you are interested in a much longer explanation, please take a look here.

Seth Kroger
56,413 PointsYou're calling logNumberOfBridges() but the function doesn't return anything, so the second log has nothing to log, or undefined
.
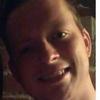
David Kanwisher
9,751 PointsWanted to add 3 examples of what they are talking about:
These will all have the desired result:
//option 1:
var Portland = {
bridges: 12,
airport: 1,
soccerTeams: 1,
logNumberOfBridges: function() {
console.log("There are " + this.bridges + " bridges in Portland!")
},
logTeams: function(){
return this.logNumberOfBridges();
}
}
Portland.logTeams();
//option 2:
var Portland = {
bridges: 12,
airport: 1,
soccerTeams: 1,
logNumberOfBridges: function() {
return "There are " + this.bridges + " bridges in Portland!"
},
logTeams: function(){
console.log(this.logNumberOfBridges());
}
}
Portland.logTeams();
//option 3
var Portland = {
bridges: 12,
airport: 1,
soccerTeams: 1,
logNumberOfBridges: function() {
return "There are " + this.bridges + " bridges in Portland!"
},
logTeams: function(){
return this.logNumberOfBridges();
}
}
console.log(Portland.logTeams());
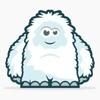
ywang04
6,762 PointsYou can just simply print out There are 12 bridges in Portland! using the following way:
var Portland = {
bridges: 12,
airport: 1,
soccerTeams: 1,
logNumberOfBridges: function() {
console.log("There are " + this.bridges + " bridges in Portland!")
},
logTeams: function(){
this.logNumberOfBridges(); //print out the message directly without console.log.
}
}
Portland.logTeams();
Harsh Khandelwal
5,539 PointsHarsh Khandelwal
5,539 PointsThanks Dino :)