Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial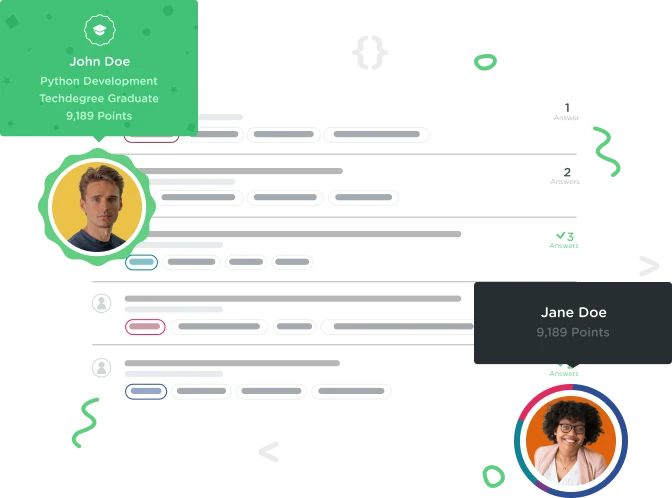

Manuel Klettke
25,324 PointsCan't seem to get the challenge "applying a discount code" working
When checking it tells me that task 1 (which was supposed to return the String discountCode in uppercase) is no longer working. In my code, as long as it doesn't throw an exception the last 2 lines should still be run, right!?
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
private String normalizeDiscountCode(String discountCode) {
int i = 0;
for (char letter : discountCode.toCharArray()) {
letter = discountCode.charAt(i);
if(discountCode.indexOf(letter) == -1 || letter != '$') {
throw new IllegalArgumentException ("Invalid discount code");
}
i+=1;
}
this.discountCode = discountCode.toUpperCase();
return this.discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer

Wan Faruq Adiputra
2,938 PointsGreetings.
From my understanding, yes as long as it does't throw an exception, the next lines will all run including the last two lines. Because if the code in a method() already throw an error, it would be inefficient to run the rest of the code in the method().
I think the reason task 1 is no longer working is because you add the code this.discountCode = discountCode.toUpperCase()
in the normalizeDiscountCode()
method.
Since you should code about this.discountCode
, only in the applyDiscountCode()
method (from task one). It is redundant don't you think applying this.discountCode
value twice, one in applyDiscountCode()
and another in normalizeDiscountCode()
.
For the if statement you should use operator (and) &&, not operator (or) ||. Since if letter
is both not a character and not a '$' symbol, then exception is thrown.
In your code, discountCode.indexOf(letter)
should always return a value more than -1. Since it is only to determined the position of letter
in the String discountCode
. -1 if it does not exist, more if it does. Since you already assign the value from String discountCode
to letter
, discountCode.indexOf(letter)
will always > -1. However this is not the code to determine if discountCode only contains characters (abc).
You should have initialized letter
as a Character class, Character letter;
. Then you could replace discountCode.indexOf(letter) == -1
to !Character.isLetter(letter)
. The method Character.isLetter(letter)
test if letter
is a character(abc).
I believe you are confused with, (for loop) for(i=0;i=MAX;i++){}
and (for each loop) for(letter: letters){}
. Since you seems like trying to apply both at the same time ;) .Craig did a workshop on this: https://teamtreehouse.com/library/feeling-loopy-with-java.
Hope this helps.
Manuel Klettke
25,324 PointsManuel Klettke
25,324 PointsThank you, got it working :)