Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial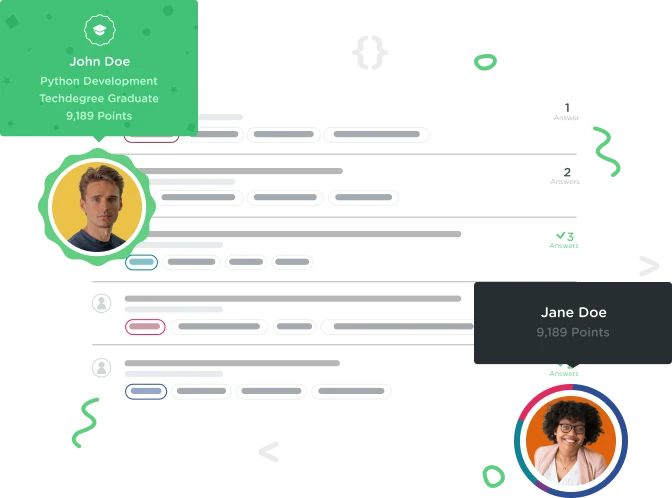

Jeremy Settlemyer
4,149 Pointschallenge very challenging
On the time_machine function challenge:
I have been working on this problem for many hours trying to get it to pass. My most recent attempts ended with f() missing x required positional argument: 'x'.
I have a funny feeling that there is something small just around the corner that I am missing.
So for the actual problem it has us take in a string.
With some testing and getting the challenge wrong it looks like there is actual strings in the string that is passed. The code I used to check for that and remove them is:
strin = strin.replace(' ', '').split(',')
for item in strin:
try:
item = int(item)
except ValueError:
strin.remove(item)
The next part was to convert everything remaining in the string to an integer that could be converted into a timedelta
here is that section of code:
for var in range(0, len(strin)):
strin[var] = int(strin[var])
Now the final and lengthy part of converting to a timedelta. I checked to see the length of the string and then made the changes based off how many variables were sent. Here is that code:
if len(strin) == 4:
delta = datetime.timedelta(minutes = string[0],
hours = strin[1],
days = (365 * strin[3]) + strin[2]
)
elif len(strin) == 3:
delta = datetime.timedelta(minutes = strin[0],
hours = strin[1],
days = strin[2]
)
elif len(strin) == 2:
delta = datetime.timedelta(minutes = strin[0],
hours = strin[1]
)
elif len(strin) == 1:
delta = datetime.timedelta(minutes = strin[0])
else:
print("You entered a invalid sequence.")
return time_machine()
finally to return the final value:
return start + delta
I have no idea of what I am doing wrong in this challenge. Any help would be great.
4 Answers
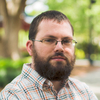
Kenneth Love
Treehouse Guest TeacherHey Jeremy Settlemyer, can you post your entire script? I have a feeling you're doing way more work than you need to. :) Sadly, it's a problem we all run into.
The challenge is quite a bit like the one before it, where you had to make a new datetime
that's a given number of hours ahead of the starter
on. The difference here is that it may not be hours that you need to advance, but days, minutes, or even years. Most of the solution relies on some if
and elif
conditions. And, of course, the tricky thing of dealing with years when you can create a timedelta
for years directly.

Jeremy Settlemyer
4,149 Pointsdef time_machine(start, string):
string = string.replace(' ', '').split(',')
for item in string:
try:
item = int(item)
except ValueError:
string.remove(item)
for var in range(0, len(string)):
string[var] = int(string[var])
if len(string) == 4:
delta = datetime.timedelta(minutes = string[0],
hours = string[1],
days = (365 * string[3]) + string[2]
)
elif len(string) == 3:
delta = datetime.timedelta(minutes = string[0],
hours = string[1],
days = string[2]
)
elif len(string) == 2:
delta = datetime.timedelta(minutes = string[0],
hours = string[1]
)
elif len(string) == 1:
delta = datetime.timedelta(minutes = string[0])
else:
print("You entered a invalid sequence.")
break
return start + delta
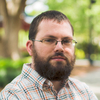
Kenneth Love
Treehouse Guest TeacherWow, yeah, way more code than you need and in completely the wrong direction.
The function you need to write takes two arguments, a number and a unit. The unit will be one of "hours", "minutes", "days", or "years. You need to return a new datetime
that is that number of that units from the starter
datetime
that's already provided.
So if your function gets 5
and "minutes"
as the arguments, it should give back a datetime
that's 5 minutes later than starter
.
Does that give you a better idea of how to do this?

Jeremy Settlemyer
4,149 PointsI had a feeling I was making it way more complicated than what it should be. At least it was good practice in taking a string and removing all the strings and keeping the integers. I will give this new idea a shot.

Jeremy Settlemyer
4,149 PointsThank you for the clarification it worked on the first try. Amazing what a different perspective will do.
Geoff Parsons
11,679 PointsGeoff Parsons
11,679 PointsHey Jeremy Settlemyer, I updated your post to use code formatting. There's a great how-to guide for using markdown within posts on the forums if you want to check it out.