Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial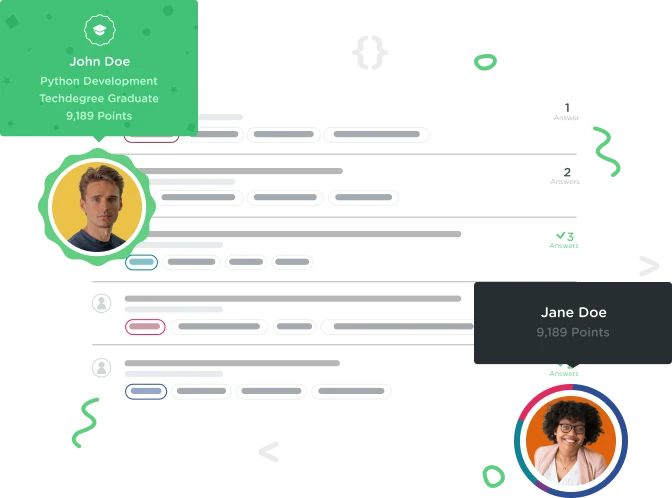

Lindsey Mote
Data Analysis Techdegree Student 1,148 PointsCode Challenge Help Please (Product Suggester)
I feel like I missed a video or two in this sequence because this assignment has been a big stretch. I went back and rewatched videos, but still struggling with comprehension. Here is the code I have and I'm still getting errors. Suggestions welcome!
def suggest(product_idea):
if len(product_idea) < 3:
raise ValueError("Please suggest an idea with more than 3 characters")
return product_idea + "mixer"
try:
new_idea = float(input("What is your new idea? "))
idea = suggest(new_idea)
except ValueError as err:
print("Oh no! Your idea needs more characters. Try again.")
print("({})".format(err))
else:
idea = suggest(new_idea)
print("{}, that's an excellent idea!".format(idea))
3 Answers

jb30
44,806 PointsIs this the challenge that you are trying to solve? If so, the return line should be return product_idea + "inator"
instead of return product_idea + "mixer"
. With that change, the suggest
function by itself should pass the challenge. The challenge does not ask for user input or for a try-except block, so those could confuse the checker and should be removed.
In the line new_idea = float(input("What is your new idea? "))
, if the user inputs the word red
, then new_idea
will be float("red")
, which gives a ValueError, since "red"
can't be converted to float. If the user inputs the number 100
, then new_idea
will be float("100")
, so new_idea
will be 100
. suggest(100)
will then give a TypeError, since floats do not have a length. Changing it to new_idea = input("What is your new idea? ")
would set new_idea
to a string, which can then be passed to the suggest
function without throwing a TypeError.
If you needed a try-except block, it might make more sense to change
else:
idea = suggest(new_idea)
print("{}, that's an excellent idea!".format(idea))
to
else:
print("{}, that's an excellent idea!".format(idea))
since idea
was already set in the try block if there wasn't an exception, and if there was an exception, you wouldn't want to say that it was an excellent idea.

Lindsey Mote
Data Analysis Techdegree Student 1,148 PointsThank you for your reply! I changed it to "mixer" just to differentiate my attempts for myself and should have changed it back before I posted here :) I originally had the input string but am getting an error with that as well. I simplified everything to this:
def suggest(product_idea):
if len(product_idea) < 3:
raise ValueError("Please suggest an idea with more than 3 characters")
return product_idea + "inator"
And this is my error:
FAIL: test_exception_not_raised (main.TestRaiseExecution)
Traceback (most recent call last): File "", line 27, in test_exception_not_raised AssertionError: None != 'baconinator' : I passed in 'bacon' and expected 'baconinator', but got 'None'
I don't know why I'm having such a hard time with this. I just downloaded a Python textbook and am going to try reading through that to see if it helps.

jb30
44,806 PointsWhitespace matters in python. The return line should be at the same indentation level as the if statement.
if len(product_idea) < 3:
raise ValueError("Please suggest an idea with more than 3 characters")
return product_idea + "inator"

Lindsey Mote
Data Analysis Techdegree Student 1,148 PointsGah! Thank you so much!!