Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial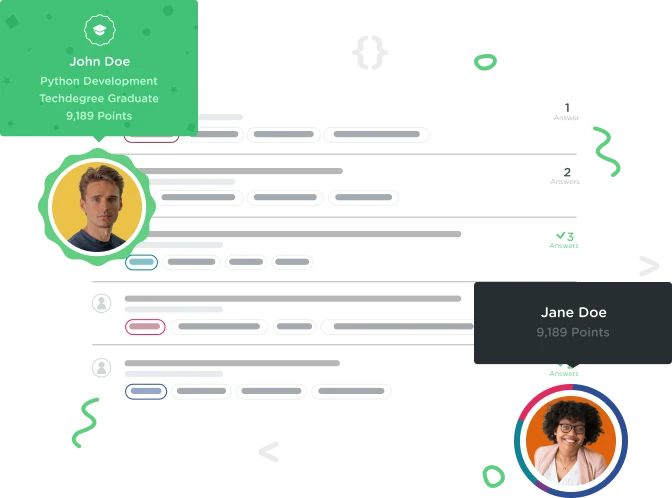
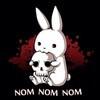
missgeekbunny
37,033 PointsCode challenge is confusing me
I cannot figure out exactly what he's trying to get us to do. It almost seems like he wants us to do the same things as the gifs but in order to create the findById type function it seems that you need to have something to loop through like how we had that gifRespository in the class. But we don't have an option or way to really create it.
package com.teamtreehouse.contactmgr.controller;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.ui.ModelMap;
@Controller
public class ContactController {
@RequestMapping("/")
public String home() {
return "index";
}
@RequestMapping("/contact")
public String contact(ModelMap modelMap) {
Contact c = new Contact();
modelMap.put("contact",c);
return "contact_detail";
}
}
package com.teamtreehouse.contactmgr.model;
public class Contact {
private int id;
private String firstName;
private String lastName;
private String email;
public Contact() {}
public Contact(int id, String firstName, String lastName, String email) {
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
public int getId() {
return id;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getEmail() {
return email;
}
public void setId(int id) {
this.id = id;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public void setEmail(String email) {
this.email = email;
}
}
9 Answers
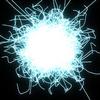
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello, I was confused on this one as well when I first took it, it's actually a lot simpler than what I thought it was, the first thing we want to do is add in the variable as a path variable, both in the constructor and the mapping request,
so it'd be something like
@RequestMapping("/contact/{id}")
Then be sure to add the path variable into the argument for the method using the PathVariable Object that we've previously used.
Then all that we are doing is passing in the value id into the parameters for the method, you still have to pass in the other string values that the constructor in your Conctact expects, but you may call them whatever you would like.
Everything else looks good.
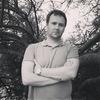
Vladimir Hisamutdinovs
11,522 PointsThis one worked for me:
package com.teamtreehouse.contactmgr.controller;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.ui.ModelMap;
@Controller public class ContactController { @RequestMapping("/") public String home() { return "index"; }
@RequestMapping("/contact") public String contactController(ModelMap modelMap) { Contact contact = new Contact(12, "one", "two", "three@four"); modelMap.put("contact", contact); return "contact_detail"; }
}

Unsubscribed User
48,988 PointsWhat's wrong with this, i really don't get it !?
@RequestMapping("/contact/{id}")
public String contactDetail(@PathVariable int id, ModelMap modelMap) {
Contact c = new Contact(12, "Max","Musterman","musterman@email.com");
modelMap.put("contact", c);
return "contact_detail";
}
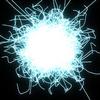
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Jens, pass in your int variable that we mapped into the constructor instead of hardcoding 12, and this should pass.

james white
78,399 PointsHi Rob,
At last you've decided to provide some English language clarity
(replace hard coded '12' with int variable),
which pointed me (and hopefully Jens) towards this code:
@RequestMapping("/contact/{id}")
public String contactDetail(@PathVariable int id, ModelMap modelMap) {
Contact c = new Contact(id, "Max","Musterman","musterman@email.com");
modelMap.put("contact", c);
return "contact_detail";
}

Unsubscribed User
48,988 PointsStill not working :-(
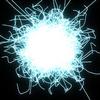
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHi Justin I understand that this is confusing, I'll see if I can explain better. Our contact item takes about 4 paraetets, one being an int and 3 being a string. So to pass the variable that we mapped into the constructor would look something like.
Contact contact = new Contact(id, "","","")
Changing the strings to whatever value that we want all that this challenge expects is to use the int value that we mapped when we create a new contCt object,
The course is new so we won't see a lot of questions about this but just keep doing what you're doing and we'll help you work out anything else needed.

james white
78,399 PointsRob's answer totally confused me...
Adding the suggested @Request line by itself just gives 3 compiler errors.
I try to google the following statement and don't get any relevant code samples:
"then once we have these two set up we can pass in the PathVariable (which we should be calling id) into the constructor. "
Just reading this statement over and over I get no hint at all
as to what type of code is needed to answer the challenge question.
So I went back to the video and at time index 02:43 there is some code like:
@PathVariable String name
...so maybe transposing "id" for name, means using something like:
@PathVariable String id
So I tried this:
@RequestMapping("/contact/{id}")
public String contact(@PathVariable String id, ModelMap modelMap) {
}
And it came up with a compiler error about missing return
so I changed the code to this:
@RequestMapping("/contact/{id}")
public String contact(@PathVariable String id, ModelMap modelMap) {
return "contactID";
}
this gives the Bummer:
Bummer! Are you sure that you returned the correct view name?
Have no idea what "view name" it wants, so stopped there.
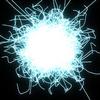
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey James you have the path variable in the request mapping set up correctly, as well as the path variable, all that you need to do now is is pass the id into the parameter in the constructor for contact, as well as the strings that it takes.

james white
78,399 PointsHi Rob,
It sounds like you are saying I'm on the right track (which is encouraging), but
"pass the id into the parameter in the constructor for contact, as well as the strings that it takes."
..sounds to me like:
Οἱ δὲ Φοίνιϰες οὗτοι οἱ σὺν Κάδμῳ ἀπιϰόμενοι.. ἐσήγαγον διδασϰάλια ἐς τοὺς ῞Ελληνας ϰαὶ δὴ ϰαὶ γράμματα, οὐϰ ἐόντα πρὶν ῞Ελλησι ὡς ἐμοὶ δοϰέειν, πρῶτα μὲν τοῖσι ϰαὶ ἅπαντες χρέωνται Φοίνιϰες· μετὰ δὲ χρόνου προβαίνοντος ἅμα τῇ ϕωνῇ μετέβαλον ϰαὶ τὸν ϱυϑμὸν τῶν γραμμάτων. Περιοίϰεον δέ σϕεας τὰ πολλὰ τῶν χώρων τοῦτον τὸν χρόνον ῾Ελλήνων ῎Ιωνες· οἳ παραλαβόντες διδαχῇ παρὰ τῶν Φοινίϰων τὰ γράμματα, μεταρρυϑμίσαντές σϕεων ὀλίγα ἐχρέωντο, χρεώμενοι δὲ ἐϕάτισαν, ὥσπερ ϰαὶ τὸ δίϰαιον ἔϕερε ἐσαγαγόντων Φοινίϰων ἐς τὴν ῾Ελλάδα, ϕοινιϰήια ϰεϰλῆσϑαι.
That is - it's all Greek to me what you are saying (and your answer doesn't really explain the "view name" error I got).
So right now I'm researching the Thymeleaf documentation and any samples I can find scattered through the internet to see if I can find enough samples to detect the pattern of what is going on with the code.
But googling for "Spring Thymeleaf example" brings up 73,700 results --it could be a while to get through all of them...
By the way this is the only forum thread for this URI challenge
https://teamtreehouse.com/community/code-challenge:11292
...in the "7922" ("Modeling, Storing, and Presenting Data") forum section for this course:
https://teamtreehouse.com/community/stage:7922
Also..it's interesting that I don't see any Treehouse forum threads for the challenges in the final section of the course "Using the MVC Architecture" when I look under all forum threads for Spring Basics:
https://teamtreehouse.com/community/syllabus:1992
That says to me either:
1.) The final section is so much easier then the ("Modeling, Storing, and Presenting Data") forum section
that no questions need be asked (unlikely),
OR
2.) Not a whole lot of people (except the very smartest who don't need to ask questions) have gotten past the Modeling, Storing, and Presenting Data challenges (and most are therefore probably "stuck" in that middle section of challenges).
In the final "Using the MVC Architecture" section, there are three "Fix" challenges --I got through the first one, but I'm stuck on the last two (they are cumulative and sequential in building one set of code atop another).
So I'm also hoping (long term) that once enough people become "unstuck" on the M.S.P.D. challenges it will break any "logjam" potentially restricting questions on the last two challenges for the course.
Personally I don't like to throw up too many threads at once - especially for courses that deal with subject matter that has never been part of a treehouse course before (Thymeleaf views).
A google search for "treehouse thymeleaf" shows that at least 3 people have gotten "achievements" for completing the course:
CJ Marchione, Saleh Jarad, and kouroshraeen
Maybe one of them will stop by to share their insights...

Unsubscribed User
48,988 Pointsno not working, is there a special connotation to it, like {} ? by the way this line was missing: import org.springframework.web.bind.annotation.PathVariable; but still doesn't work.....

Unsubscribed User
48,988 Pointsno @PathVariable needed.... past the challenge without it.....

Robert eRS
9,646 Points@RequestMapping("/contact/{id}") public String contact(@PathVariable int id, ModelMap modelMap) { Contact c = new Contact(id ,"","",""); modelMap.put("contact",c); return "contact_detail"; }
missgeekbunny
37,033 Pointsmissgeekbunny
37,033 PointsThe code that's there is the code in the challenge when you first start . . .
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHi You are correct, it is, and it's all going to be used, we just need to add a few things to it, mostly pass in the variable into our @RequestMapping item, then add in a path variable, then once we have these two set up we can pass in the PathVariable (which we should be calling id) into the constructor.
Is there something I could explain better, I don't want you think there's any of that code that needs to be deleted, just added to.
missgeekbunny
37,033 Pointsmissgeekbunny
37,033 PointsI was just making sure you understood that I didn't write any of that.
missgeekbunny
37,033 Pointsmissgeekbunny
37,033 PointsNow that I've had a chance to actually sit down and finish the challenge, I'm through to the next part. Thanks for explaining what he was looking for to me.
Justan Human
71,770 PointsJustan Human
71,770 PointsHow do you passing in the value id into the parameters for the method?
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Justin we just need to pass that parameter in like we were creating a new Contact object. And where the constructor asks for an int just use the value that we have mapped for it. And whatever strings we would like.