Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial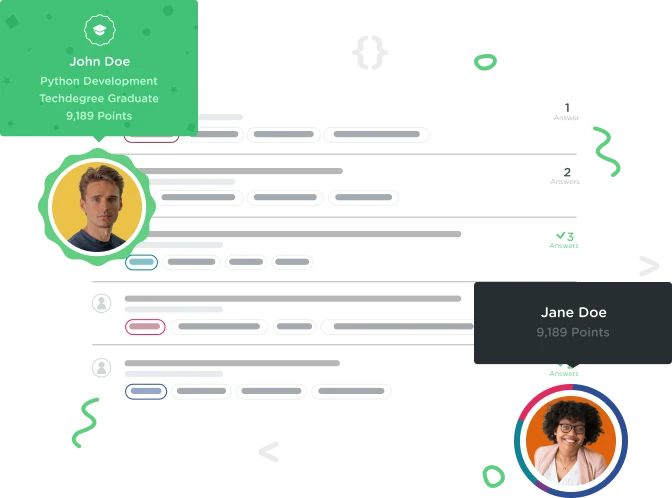

Min Kwang Ng
17,743 PointsCode works on workspaces but getting "Can't get the length of a 'Hand'" error
For the rpg-roller code challenge, I am getting the error "Can't get the length of a 'Hand'". However when I run the same code in workspaces I do get the correct value for len(h).
My implementation is
from dice import D20
class Hand(list):
@property
def total(self):
return sum(self)
def __init__(self):
super().__init__()
def roll(self, size):
for _ in range(size):
self.append(D20())
When I run this
>>> import hands
>>> h = hands.Hand()
>>> h
[]
>>> h.roll(2)
>>> h
[15, 7]
>>> len(h)
2
>>> h.total
22
This seems to be what the challenge is asking for. What am I missing from my implementation?
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
def __repr__(self):
return str(self.value)
class D20(Die):
def __init__(self):
super().__init__(sides=20)
from dice import D20
class Hand(list):
@property
def total(self):
return sum(self)
def __init__(self):
super().__init__()
def roll(self, size):
for _ in range(size):
self.append(D20())
[MOD: added ```python formatting -cf]
3 Answers

ds1
7,627 PointsThanks, Steven- your answer was helpful!
Min, I pretty much did the same thing as you... checked it and it worked outside of the exercise... couldn't figure out what was going on till after I read this and played around with it ALOT... thanks for posting the question. My solution FINALLY passed after I made roll() into a class method that created an instance of Hand(), did the append() with D20() to this instance, and returned the instance.
I guess the clue that I should have used a class method is the "Hand.roll..." in the question. Totally missed that- I just thought that 'Hand' was a placeholder for a Hand instance. I assumed that the check would do something like:
h = Hand()
h.roll(2)
[etc.]
instead of.....
Hand.roll(2)
[etc.]
A hint to use a class method in the instructions could be really helpful! FYI Chris Freeman in case you deem it a worthy revision. Thanks, D.
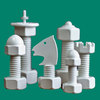
Steven Parker
231,264 Points
Be careful about testing a challenge in an external REPL.
If you have misunderstood the challenge, it's also very likely that you will misinterpret the results.
In this case, the challenge is expecting roll to return an instance of Hand, but your method doesn't return anything.
Another hint: since you're creating a new instance inside the method, this might be a good use for a classmethod or a "staticmethod".

ds1
7,627 PointsOh, Ok.. thanks for your help, Chris!
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsA
@classmethod
isn't explicitly required. A@staticmethod
that doesn't usecls
orself
as its first parameter would also work.A solution could have
Hand.__init__
take acount
argument for how many D20 die to initiate. Then have a static methodroll
pass the count value to the init and returnHand(count=count)
.ds1, feel free to also tag Kenneth Love directly when you have a suggestion to improve a challenge.