Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial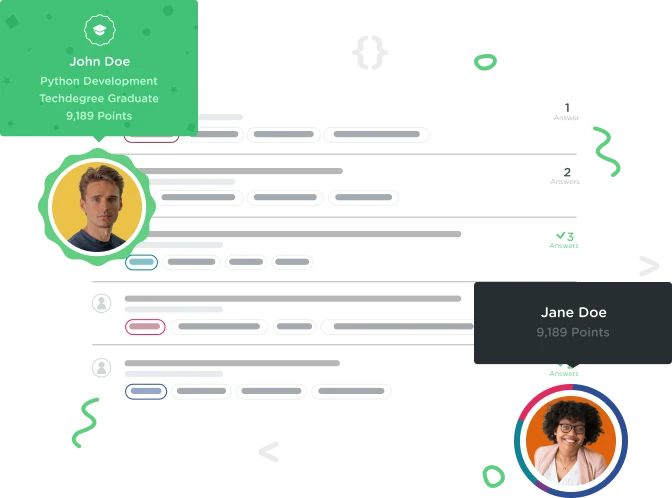

Miguel Matos
6,365 PointsCompletely lost with this challenge - Inheritance
The Polygon class represents a 2 dimensional shape. It has a public field named NumSides. Create a new type of polygon called Square. It should have a public readonly field named SideLength that’s initialized using the constructor. Use base to initialize NumSides to 4.
That is the challenge and I cannot figure out what to do. In base if I enter 4 I get a message saying: "Oh no!
There was a temporary issue running this code challenge. We've been notified and will investigate the problem.
If you continue experiencing problems, please contact support at help@teamtreehouse.com."
if I change the number 4 in base to numSides it tells me "Did you initialize the field 'numSides' to 4 in the base constructor?"
I am completely lost at how to make it work. I rewatched the video multiple times and googled base c# but still cannot solve this.
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
}
12 Answers

Miguel Matos
6,365 PointsPlease stop responding guys lol. I figured the answer out by myself a long time ago. It was a bracket placement error
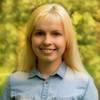
Iryna Bondarenko
777 PointsHi Miguel! Probably, you need to create Square class outside the Polygon class. It should solve the problem.

Miguel Matos
6,365 PointsI literally just figured out the problem... I put the closing bracket in the wrong spot on accident. I was struggling over it for 3 hours. Well the nightmare is over and I have learned an important lesson in bracket management. Thank you for your reply

Andy Hammond
712 Pointsokay, this worked :P Its crazy how simple some solutions can be, yet I tend to complicate things....is this normal? :P
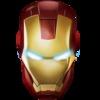
Aaron Campbell
16,267 PointsJust make another class in the same file (remember you can have multiple classes in one file!) called Square and have it extend Polygon. From there, you can initialize the SideLength variable:
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4){
SideLength = sideLength;
}
}
}

Norbu Wangdi
7,187 Pointscan somebody help me, whats wrong with my code
namespace Treehouse.CodeChallenges { class Polygon { public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
class Polygon : Square
{
public readonly int SideLength;
public Square(int sideLength) : base(4);
{
SideLength = sideLength;
}
}
}
}
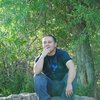
Brody Ricketts
15,612 PointsSquare is a subclass of Polygon. Polygon is not a subclass of Square.
Specifically, your issue is right here:
class Polygon : Square
Consider revising the order of those two.

Chris Gallego
7,231 PointsCan someone please tell me the reason why //NumSides is capitalized when it is first declared, and then changed to lowercase //numSides in the constructor? It's the same with //SideLength and //sideLength. Thank you!

Edward O'Connor
9,000 Pointsyou also need to get rid of the semi-colon at the end of this line
public Square(int sideLength) : base(4);
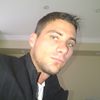
John Lukacs
26,806 Pointsnamespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square: Polygon {
public readonly int SideLength;
public Square(int SideLength):base 4
{
SideLength = sideLength;
}
}
where did I go wrong for this challenge

Abubakar Gataev
2,226 PointsThe S of sideLength should be s
public Square(int SideLength):base 4
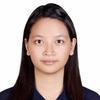
Yi Chiang
11,738 PointsAccording to Video, I think the answer should be class Square : Polygon. I think that your solution is correct. ''' class Square : Polygon { public readonly int SideLength; public Square(int sideLength) : base(4) { SideLength = sideLength; } '''
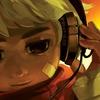
Jamal Scott
9,656 Pointspublic Square(int sideLength) : base(4);
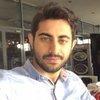
HIDAYATULLAH ARGHANDABI
21,058 Pointsnamespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4){
SideLength = sideLength;
}
}
}
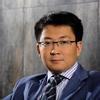
Andrei Li
34,475 PointsThis is a correct solution.
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
}
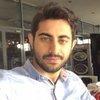
HIDAYATULLAH ARGHANDABI
21,058 Points```namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
}
Brody Ricketts
15,612 PointsBrody Ricketts
15,612 PointsYou shouldn't ask people to stop responding to your post. Disable notification on your post if you are tired of the email notifications. Other Treehouse Student's might have this EXACT question and rather than asking a brand new question, they can find the answer here.
Good luck and Happy coding.