Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial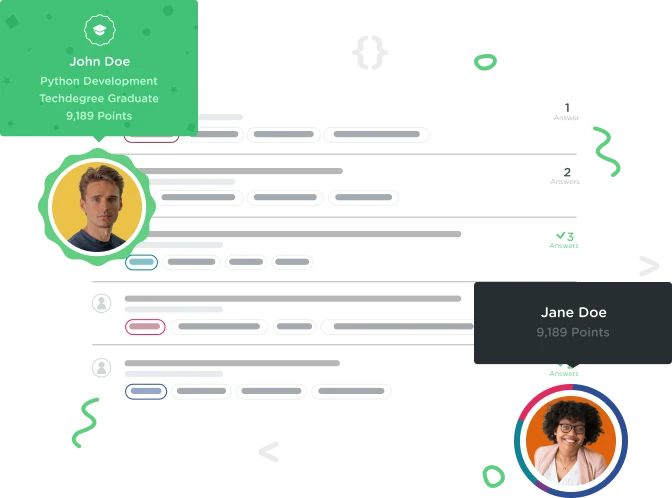

Diane D
800 PointsConfused by the fizz buzz challenge and the if, else, elif, statements in python
To whom this gets read by, I just finished the python challenge regrading comparison operations and I am pretty confused. This is what I don't understand about the else, if, and elif functions;
1) what exactly are we saying with these three functions? I understand that they are a form of logic, but it doesn't make much sense to me of how and when they are used.
2) Are the else, if, and elif statements the only lines of code that we indent in the format below and why? example; else: print("is neither a fizz or buzz number.")
3) And what does the {}! and .format( , )) mean in these print functions while using if, elif, and else? For example, what are we really saying in this print function and why do we format it like that? example; print("Hey {}!\n The number {}...".format(name, number))
I greatly apologize if this question is a bit much, but I have been struggling for days on this topic and have been unable to figure it out on my own. Any help on any of this is much appreciated!
2 Answers
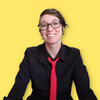
Mel Rumsey
Treehouse ModeratorHi Diane D! I am glad you asked, these are going to be concepts that you use a lot so understanding why and when to use them is very important. :)
Alright to explain if, else, elif:
Let's say you are building a program that you are wanting to greet the user by name.
name = input("What is your name?") # this will ask the user what their name is and store it into a name variable
# then we want to determine how to respond based on their input
if name == "Diane":
print("Welcome back, {}!".format(name)) # It will ONLY respond with this *IF* the user types "Diane", if not it moves
#down the list to the next if
elif name == "Mel":
print("What's up, {}!".format(name)) # elif will then get called if the user does not type Diane. But it will only
#print this message if the user types "Mel"
else:
print("Nice to meet you, {}.".format(name)) # If the user does not time in Diane OR Mel, this line will run with the
# name that they enter
If, elif, and else (always in this order) narrows down what line of code to execute based on what is true.
1.) If the user types in Bob, the program runs and asks "Is this name equal to "Diane"? no
2.) Okay next... "Is this name equal to "Mel"? no
3.) Okay next... well, no more conditions so this one is true. "Nice to meet you, Bob." will then print to the console.
The indenting is used in other parts of python too, like functions, classes, and methods, but you will get to those soon.
*and .format(): *
The .format() method is a way of using different variables throughout a string. Using {} as placeholders throughout the string and then declaring what will be filled into those spots makes the code more versatile and less hard coded. The name variable can keep being used, but the value of the name variable can change.
If you wrote out,
print("Welcome back, Diane!")
You have no option to change it later without changing the line of code again and again. With the format method, you can insert variables into strings.
Hopefully this example helped, if not let us know! Asking questions are great practice, keep them coming! :) We are always happy to help! 💚💚💚

Diane D
800 PointsThank you guys for your help and input! That makes a lot more sense now.
Tiffany Greathead
6,613 PointsTiffany Greathead
6,613 Points1) The purpose of if, elif and else statements is to execute outcomes only if they meet certain conditions. For example, if you're building a program that tests elementary school students on their math skills, for the question, "2 + 2 = ?", IF their answer is 4, you'd want to print "That's correct!" Else: (if their answer does not equal 4) print, "That's the wrong answer.
2) Python uses whitespace and indentation to structure their code. In the case of if, elif, else, you indent to indicate you only want something to happen if it meets a certain condition. In the above example, print("That is the right answer") is indented after the if statement because I only want to tell the user that their answer is right if it equals the integer 4. If I didn't indent print("That is the wrong answer") under the else statement, then the program would print "That is the wrong answer" even if the user entered the right answer.
You can read more about whitespace here: https://stackoverflow.com/questions/13884499/what-is-python-whitespace-and-how-does-it-work.
3) In python, there's different ways to print variables in a string. .format() is one example and it's not just used for if, elif and else statements, but anytime you want to print a variable in a string:
The .format(variable1, variable2) is saying, for the first set of {}, print variable1, for the second set of {}, print variable2, and so on and so forth. \n in python is used to indicate a new line, kind of like an enter key on your keyboard, so if you were to copy my code in your workspace and run it in the terminal, anything after the \n is printed on the next line.
I hope this makes sense.