Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial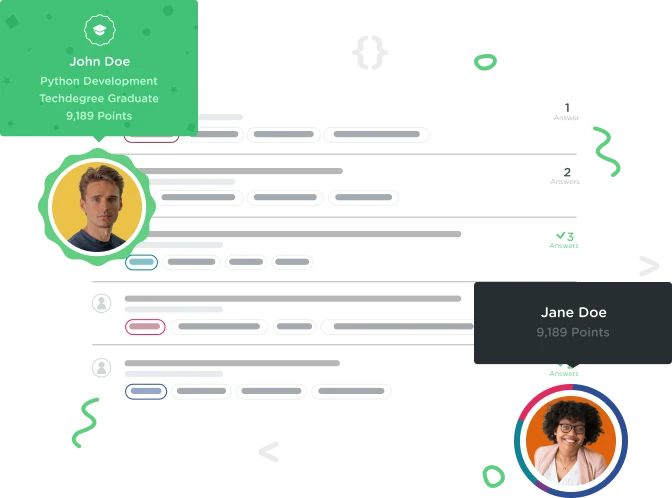
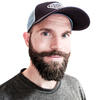
Daniel Breen
14,943 PointsConfused on Encapsulation. Should I keep stabbing at it?
I'm a little confused about encapsulation. I understand the point and what to do to encapsulate a field (the underscore convention). In other words, if someone tells me to encapsulate something, I know to make a private field with the underscore and then a public property without the underscore.
I'm 75% there. I can't even really put into words the part that I don't understand. It just looks kinda weird to me.
If I understand this much, will I just have one of those "aha!" moments further down, or is it something I should go over a few times until I have a better grasp?
1 Answer

Pirvan Marian
14,609 PointsI am not into C# but I'll do my best to explan how I understand it.
Encapsulation is one of the fundamental principles of the OOP style.Protect your properties from outside access and allow outside access to them using methods.
Remember, properties store data and methods are there to interact with the properties, with the data.This interaction is made only by methods to assure your class is well structured.
When I use the term "outside", basically it means outside the class/object.
Why you would do something like this? Because someone, even you, can change the normal flow of a class by setting directly a value for a property, a value that breaks everything.
Let me give you a more clear example:
You have a class called Money.This class has a public property called amount and two methods: getAmount() and setAmount(amount).The first method returns the content of the amount property and the second one set the content of the amount property.The second method has some logic too, it checks if the amount is not a negative value, so we can't store negative values.
Great, thins are great until now, but we have one problem, the property amount has public visibilty, basically it means that I can directly modify the content of the amount property.What does it mean? I can set any value I want, even a negative one.Why I can do that? Because it has public visiblity, the logic from the setAmount() method is not used anymore, you see, this is a big problem, we don't want negative values, just 0 or any other greater value.
Iam giving you an example in PHP, wich is a little similar, OOP concepts are the same no matter wich language you use.
<?php
class Money
{
public $amount = 0;
public function getAmount()
{
return $this->amount;
}
public function setAmount(integer $amount = 0)
{
// the logic to check if the amount is not a negative value
if ($amount < 0) {
throw new Exception("The amount can be a negative value");
}
$this->amount = $amount;
}
}
// we create an object for the Money class
$money = new Money;
// we set the amount to 10 by using the setAmount() method
$money->setAmount(10);
// it displays 10, great.
echo $money->getAmount();
// because the $amount property is public, we can access it directly outside the object
// look at what I do, I set a negative value,
$money->amount = - 10;
// it displays -10, I bypassed the rule without negative values.
echo $money->getAmount();
It can create tons of problems, set a different visibility for your properties and use methods to act on them.
Use methods like the one from above when interacting with properties, one gets the value of a property and one sets a value for a property.
Daniel Breen
14,943 PointsDaniel Breen
14,943 PointsOkay. That clears things up. This kind of reminds me of writing validations for my endpoints when I was working with server-side node/restify to keep the database rom getting angry. I never got much into object oriented javascript.