Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial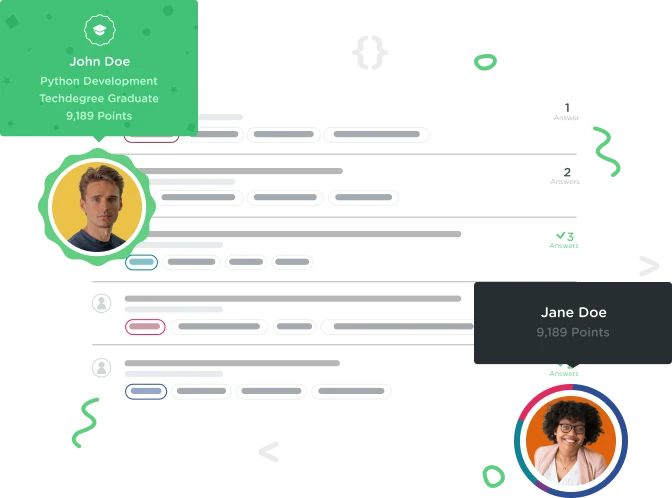

Araz kuberin
6,199 Pointscorrect the existing hasTile method to return true if the tile is in the tiles
public boolean hasTile(char tile) { boolean isHit = tiles.indexOf(tile) !=-1; if(isHit){ return true; } else { return false; } }
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
boolean isHit = tiles.indexOf(tile) !=-1;
if(isHit){
return true;
}
else {
return false;
}
}
}
}
4 Answers
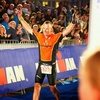
Steve Hunter
57,712 PointsHiya,
Try using indexOf(tile) > 0
to indicate that tile
is inside tiles
. Once you've done that, you can just return the result of that comparison; you don't need the if
conditional.
Try:
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
return tiles.indexOf(tile) > 0;
}
Make sense?
Steve.

Araz kuberin
6,199 Pointspublic class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
boolean isHit = tiles.indexOf(tile) !=-1);
if(isHit){
return true;
}
else {
return false;
}
}
}
}
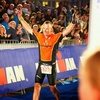
Steve Hunter
57,712 PointsThere is an issue with your brackets. Where you are declaring the (unnecessary) boolean
called isHit
, you have closed a bracket behind the -1
but never opened them.
As in my previous answer, you don't need to do all this leg work to pass the challenge.
You want to check that the tile
is in tiles
. To do that, check that the tiles.indexOf(tile)
is greater than zero. That check will produce a true or false answer. That is the boolean
you want to return from the hasTile
method. So, inside hasTile
you only need one line of code, as above.
Let me know how you get on.
Steve.

Araz kuberin
6,199 PointsOK. i have tried so many ways could you please give me one code that can pass that exercise.
This didnt also work.
public boolean hasTile(char tile) {
return hasTile.indexOf(tile) >=0; }
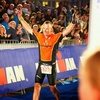
Steve Hunter
57,712 PointsI have given you the code to complete the challenge! If you look at what you've written, it isn't quite the same as what I have suggested.
You can't call hasTile
inside itself. So, change your line:
return hasTile.indexOf(tile) >=0;
to call indexOf()
on tiles
. As above, the entire method will look like:
public boolean hasTile(char tile) {
return tiles.indexOf(tile) > 0;
}
Let me know how you get on.
Steve.

Araz kuberin
6,199 PointsThank you! it worked now. I also recognised that i had a extra brackets in some of my last codes and i think i missed that many times as well! I copy pasted your code and that gave me still error untill i recognized there is a extra brackets. but in many of my code it was not only the extra brackets causing the problem i think it was more things that i missed!
Araz kuberin
6,199 PointsAraz kuberin
6,199 PointsHello,
thank you! i still cant pass!
./ScrabblePlayer.java:23: error: class, interface, or enum expected } ^ Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 1 error
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsCan you post all your code so I can replicate the error.
Thanks,
Steve.