Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial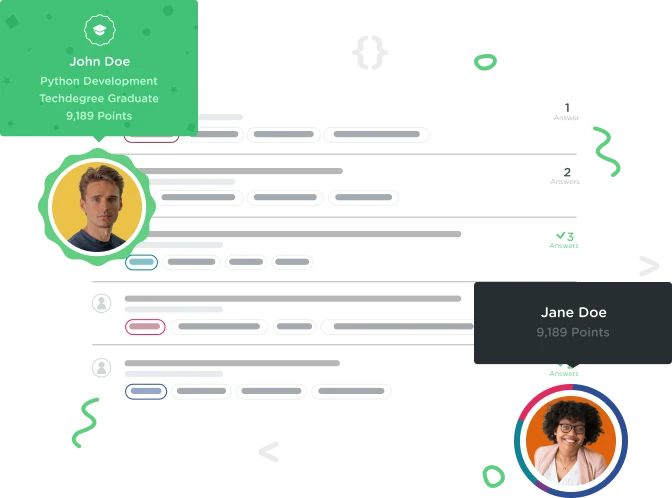
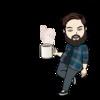
Philip Schultz
11,437 PointsCould I clean this up?
Hello, Is this how I should have solved this problem? Does anyone have any suggestion on how I could have approached this challenge differently? Is there anything in my code that shouldn't be there or could be more efficient?
def combiner(arg):
word =''
num = 0
for item in arg:
if isinstance(item, int):
num += item
elif isinstance(item, float):
num += item
elif isinstance(item, str):
word += item
return word + str(num)
2 Answers
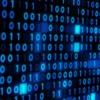
Alexander Davison
65,469 PointsYour answer is fine, but if are nit-picky about simplicity and clarity, you might write this instead:
def combiner(arg):
string = ''
num = 0
for item in arg:
item_type = type(item)
if item_type in (int, float):
num += item
elif item_type == str:
string += item
return string + str(num)
This is only a little bit cleaner. I would probably write something like the above as my solution.
If you are going for minimal lines of code, you can (but probably shouldn't) write:
from functools import reduce
def combiner(list):
string = reduce(lambda x, y: x + (y if isinstance(y, str) else ''), list, '')
sum = reduce(lambda x, y: x + (y if isinstance(y, int) or isinstance(y, int) else 0), list, 0)
return string + str(sum)
This is concise, but who would want to debug that? (In fact, there actually is a bug! It's nearly impossible to spot.)
I hope this helps! ~Alex

Wenye Zhao
2,011 PointsOne truncation I can can suggest is combining you two elif
statements for int and float:
def combiner(arg):
word = ''
num = 0
for item in arg:
if isinstance(item, str):
word += item
elif isinstance(item, (int, float)):
num += item
return word + str(num)