Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial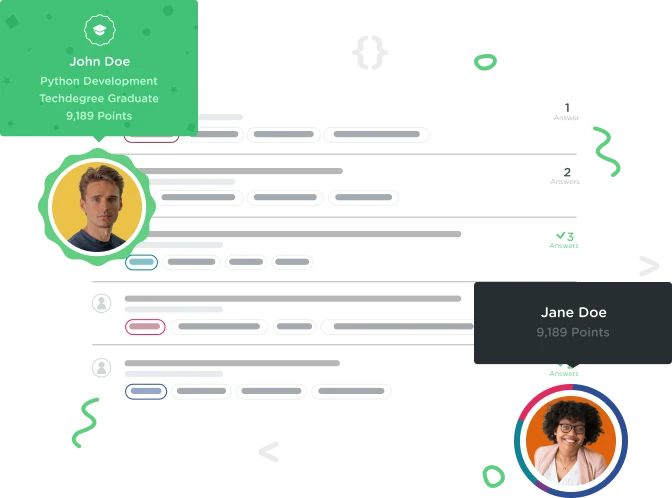
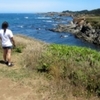
Nancy Melucci
Courses Plus Student 36,143 PointsDeclaring variables as decimals, parsing user input as Int32 - is this really a problem?
I recently took an exam in C# in an on the ground class. A simple batting average program. I declared my variables as decimals and then parsed the user input as Int32. I ran the program about a dozen times before submitting it for grading. It ran, completed, the output was identical to the printout provided by the instructor. The program exited with code 0.
I got my grade and I was docked 1/3 of available points and told that my program "terminated" because of the decimal variables/int parse described above.
I thought it was a problem to declared integers and then input or parse decimals - not the other way around.
Terminated is not the same as crashed. My program did not crash.
Not just venting (ok, venting a little). My question is, would declaring decimals and parsing integers really cause huge problems in real life coding? If so, how? Because my program did not have any problems running. Is the huge penalty exacted here related to an error I might commit later on as a programmer?
I would like to know. I feel awful, especially since the feedback that the program "terminated" tells me nothing. All programs terminate at some point. What gives?
Thanks.
8 Answers

William Schultz
2,926 PointsI'm still a newbie and learning, so I may be wrong in my answer. Keep that in mind. I think you got marked down as your code indicates you may not have the concept of integral and real types fully understood.
When you declare your identifiers/variables you declare them as decimal but place an integer in them, which is implicitly converting the literal integer to a decimal. (to initialize a decimal value you would use an M at the end. i.e 0.0M), but it is acceptable to use an integer and implictly convert it.
From what I see you are parsing the "string" input from the user into integers and implicitly converting them into decimal by placing the results in totalOuts and totalHits which were declared as decimal.
My question would be, why are you parsing the user input into an integer instead of into a decimal value, if you wanted to use decimal values? If you want to use decimal values, then just use decimal.Parse.
On another subject is how accurate do you need this data. Decimal is normally used for very accurate floating numbers and is used in spreadsheet, financial, and banking type of applications. For this program I think I would have just used double instead of decimal.
Hope this helps. If anyone finds my information incorrect, please let me know. I'm still a newb.
Also I would recommend you watch the "working with integers", "working with floating-point types", and "calculating a statistic" videos in the 'parsing data' section of the "C# streams and data processing" course. It will probably help a lot. https://teamtreehouse.com/library/c-streams-and-data-processing
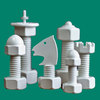
Steven Parker
231,198 PointsThe instructor may have used different data for evaluating and grading.
It could be the data provided as an example was not the same as that used for grading, so you may have overlooked a data-related functional issue.. Even the automated challenges here often do testing with more extensive data than what is provided as an example.
To make a more complete analysis possible, you'd need to supply at least the code and the conditions of the assignment, and preferably the data used for the final performance evaluation.

James Churchill
Treehouse TeacherNancy,
Steven makes a great point. I'd ask the instructor (or the person who did the grading) why you were marked down. Make it clear that you aren't challenging the decision, but rather trying to learn from the experience.
I would also add that if I was code reviewing the code that you've described, I'd be left wondering if those values were supposed to be decimals (as they are being stored in memory) or integers (as the user input is being parsed). Perhaps a code comment would clarify the intention, though it seems unusual that you'd parse user input into an integer only to store that value in a decimal variable.
Thanks ~James
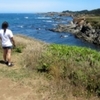
Nancy Melucci
Courses Plus Student 36,143 PointsYes. I will need to reproduce the program from memory, it was very simple and that should not be a problem (calculating a batting average). I will post the code later. I actually get another shot at this and I DO want to learn. I am curious about how large the penalty was (50 out of 150 points) given the fact that the code RAN and the program terminated with exit code 0 (that is normal, right, not crashing). So the penalty just seems...way out of proportion. And I did run the program with the instructor supplied data. It ran. Is there a difference between terminating and crashing? Can a program terminate incorrectly? The answer was right. No crash. This is the source of my confusion.
I'll post the code within the next 12 hours. Thanks.
Nancy M.
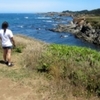
Nancy Melucci
Courses Plus Student 36,143 Pointsusing System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BattingAverage
{
class Program
{
static void Main(string[] args)
{
decimal totalAtBats = 0;
decimal totalOuts = 0;
decimal totalHits = 0;
Console.WriteLine("Enter outs: ");
totalOuts = Int32.Parse(Console.ReadLine());
Console.WriteLine("Enter hits: ");
totalHits = Int32.Parse(Console.ReadLine());
totalAtBats = totalOuts + totalHits;
decimal battingAverage = totalHits / totalAtBats;
Console.WriteLine("You had " + totalAtBats + " total at bats.");
Console.WriteLine("Your batting average is " + Math.Round(battingAverage, 3));
}
}
}
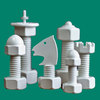
Steven Parker
231,198 PointsWhile it may not represent realistic data, giving this program an input with a decimal value does make it crash, terminating it before it would otherwise finish. This might be what the instructor was talking about.
But to be sure, you might take James's advice and ask the instructor directly.
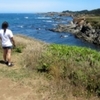
Nancy Melucci
Courses Plus Student 36,143 PointsSure. It's unlikely that hits or outs would be input as decimals in real life. But I get your point. I am waiting to hear from the professor.
I still feel as if the penalty was way out of proportion with the scale of the mistake but if I can re-do the assignment I will not carry on about it and end up dying on this hill.
thanks everyone.
NJM
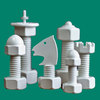
Steven Parker
231,198 PointsLet us know how it turns out and what explanation you get.
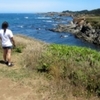
Nancy Melucci
Courses Plus Student 36,143 PointsI will. Thanks again.
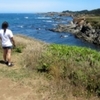
Nancy Melucci
Courses Plus Student 36,143 PointsI got my points back. In the future, I will not declare and parse in two different numeric types. I am sure that while it mattered not with this simple operation it would cause bugs in a more complex program.
thanks everyone for your help...I am grateful for this community as I struggle to be competent at coding.

James Churchill
Treehouse TeacherYou're doing great! Hang in there :)