Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial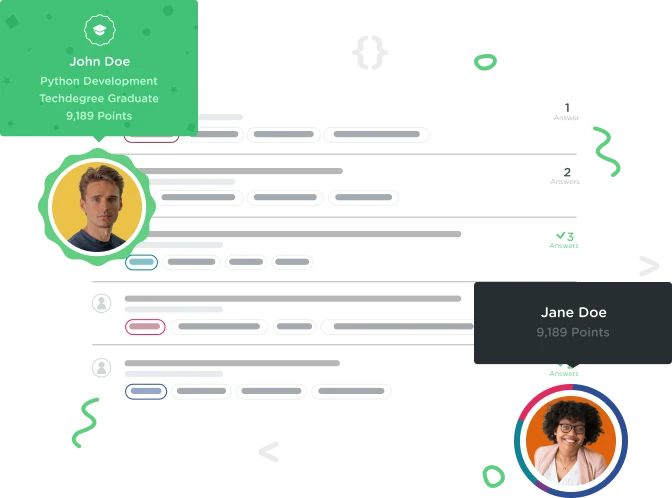
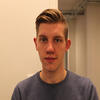
Coen van Campenhout
5,034 PointsDependancy injection in web applications
So in school we are building web applications in C# using asp.net, next year we will be using spring to do the same in java. Recently we were having a discussion about dependancy injection and what it is but none of us could really get it clear.
Is there anyone here who can explain dependancy injection or has any good information about it?
2 Answers
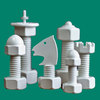
Steven Parker
231,236 PointsI saw a funny description of Dependency Injection as a "25-dollar term for a 5-cent concept".
Basically, it's just passing references to dependencies to an object constructor rather than referencing global resources or constructing them internally.
Without dependency injection:
public SomeClass() {
myObject = GlobalService.getObject(); // a library service is used
}
With dependency injection:
public SomeClass (ServiceClass theService) {
myObject = theService.getObject(); // the injected service is used
}
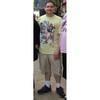
David Good
12,348 PointsYou use dependency injection to split up your codes into separate testable parts. When you instantiate an object, the constructor can have parameters that are interfaces. The dependency injection manager takes a look at what interfaces the new object is asking for and then gives it the proper objects that those interfaces represent.
public class MainClass
{
private readonly IMyService _myService;
public MainClass(IMyService myService)
{
_myService = myService;
}
}
Interface IMyService
{
public void DoSomething()
}
public class MyService : IMyService
{
public MyService()
{
}
public void DoSomething()
{
//put code to do something here
}
}
In this example we have an Interface called IMyService which tells us that a class that implements this interface has to have a void method called "DoSomething". The MyService class inherits from IMyService so it has the method DoSomething(). Now when MainClass is instantiated, it is going to ask for an object that implements the interface IMyService. In the background, your dependency injection tool (autofac, etc) would then give MainClass an instance of MyService. You could also have other classes that implement the class IMyService and inject those into the constructor in other situations.
The simplest example
public int AddThis(int a, int b)
{
return a + b;
}
In this case you injected a and b to the method AddThis.
Take a look at this for a more complex example