Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial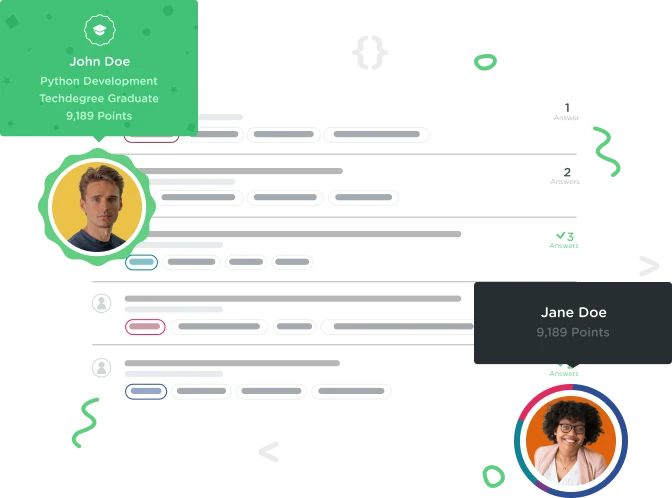

wdhr423425233
9,285 Points"Did you alter the original EatFly method instead of creating a new one?" No I didn't. I deleted it all and wrote it.
So this error won't go away. I'm getting no compiler errors. Just this message that won't go away no matter how many times I try to re-create the method. My logic is correct. Just won't let me continue.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public Frog(int tongueLength, int reactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
}
public bool EatFly(int flyReactionTime, int distanceToFly)
{
if (ReactionTime <= flyReactionTime && TongueLength >= distanceToFly)
{
return true;
}
else
{
return false;
}
}
}
}
1 Answer

andren
28,558 PointsYou get that message because you are not meant to touch the original method at all in any way. And removing it entirely certainly counts as touching it.
For challenges it's generally a bad idea to do anything not explicitly asked for in the instructions, and the instructions says nothing about removing the method. That means you have to add the original method back in order to complete the challenge.
There is another issue with your code though, which is that the instructions told you to create a method that took distanceToFly
and flyReactionTime
as parameters. You have defined the parameters in the opposite order, which will create an issue since the order of the parameters determine what arguments gets assigned to what parameter.
I would also add that your if
statement is actually pretty redundant, the expression you have written will result in true
or false
on its own and can be returned directly.
Here is the solution to the exercise:
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public Frog(int tongueLength, int reactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public bool EatFly(int distanceToFly, int flyReactionTime)
{
return ReactionTime <= flyReactionTime && TongueLength >= distanceToFly;
}
}
}
wdhr423425233
9,285 Pointswdhr423425233
9,285 PointsTHANK YOU!! I couldn't figure out what was causing the error. I started changing things and writing things different ways to try to see if it worked. But I realize it just wanted a new method with different parameters. Thanks for the explanation!
Ar33ss Leon
4,621 PointsAr33ss Leon
4,621 PointsAs far as I know you cant define two methods with the same name, I tried and it did not work. I get errors like its already exist in the code. How?