Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial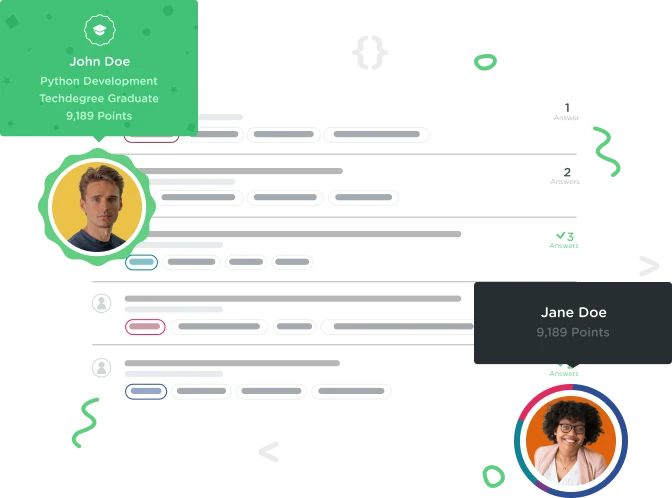

Iain Simmons
Treehouse Moderator 32,305 PointsDidn't consider Leap Day (Feb 29)?!
One thing that I noticed this task didn't consider is what happens when the user wants to view the page for February 29. It should print http://en.wikipedia.org/wiki/Feb_29
, but instead it will throw a value error, and state that it isn't a valid date.
First I tried just using the .replace
method to change the year
of the datetime object to be in a leap year (e.g. 2012), but that didn't seem to help.
The only thing I could think of (besides importing additional Python libraries to handle just this case) is to add another conditional with the check for 'quit'
to print the correct link if the user asks for February 29.
e.g. (note that I'm using a different date format because I'm from Australia):
from datetime import *
answer_format = '%d/%m'
link_format = '%b_%d'
link = 'http://en.wikipedia.org/wiki/{}'
leap_day_answer = '29/02'
leap_day_str = 'Feb_29'
while True:
answer = input("\nWhat date would you like? Please use the DD/MM format."
+ "\nEnter 'quit' to quit.\n")
if answer == leap_day_answer:
print(link.format(leap_day_str))
continue
elif answer.lower() == 'quit':
break
try:
date_obj = datetime.strptime(answer, answer_format)
output = link.format(date_obj.strftime(link_format))
print(output)
except ValueError:
print("That's not a valid date. Please try again.")
Anyone else got any ideas on how this should be handled?
Hopefully Kenneth will do a course that covers testing in Python, so we can pick up on things like this.
2 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsHere's my updated code, where you define a leap year string and the format that you will use for it:
from datetime import *
answer_format = '%d/%m'
link_format = '%b_%d'
link = 'http://en.wikipedia.org/wiki/{}'
leap_year_format = '%Y'
leap_year_str = '2012'
while True:
answer = input("\nWhat date would you like? Please use the DD/MM format."
+ "\nEnter 'quit' to quit.\n")
if answer.lower() == 'quit':
break
try:
date_obj = datetime.strptime(answer + leap_year_str, answer_format + leap_year_format)
output = link.format(date_obj.strftime(link_format))
print(output)
except ValueError:
print("That's not a valid date. Please try again.")
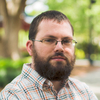
Kenneth Love
Treehouse Guest TeacherHmm, in the console, I can do datetime.date(2012, 2, 29)
and get a valid object. Some with datetime.datetime(2012, 2, 29)
. If I try the some date for 2013, I get a ValueError
as expected.
The problem in the course, of course, is that we're not specifying a year for our dates.
EDIT: And, yes, we're going to a testing course eventually. Probably after Django Basics or whatever follows that.

Iain Simmons
Treehouse Moderator 32,305 PointsOkay so I just realised of course that if you don't specify a year with strptime
, it defaults to the year 1900, which isn't a leap year.
Also, datetime.replace
returns a new datetime
object, so it won't work if the original object is invalid.
So a better solution to this issue would be to explicitly define a leap year in addition to the user's input.
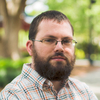
Kenneth Love
Treehouse Guest TeacherYeah, if you want it to be able to specify a leap year (which you probably do) the hard code a year that's a valid leap year.