Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial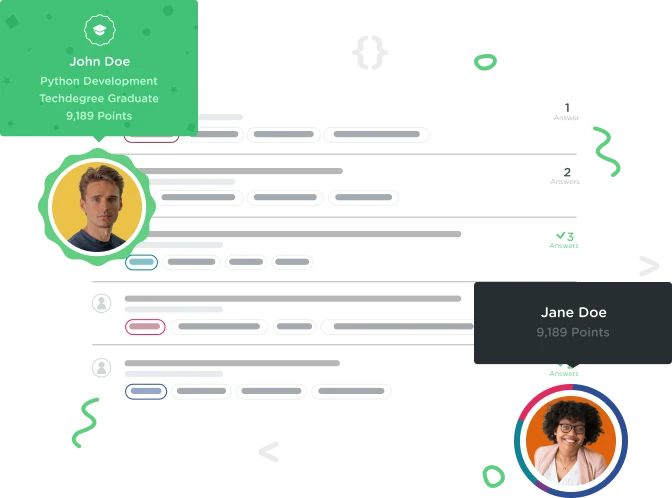
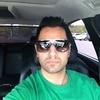
Bikram Mann
Courses Plus Student 12,647 PointsDifference between A is not None, A not None and A != None ? Are they all valid in python? Do they all yield same result
A is not None, A not None and A != None ? Are they all valid in python? Do they all yield same result ?
4 Answers
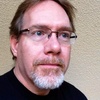
Chris Freeman
Treehouse Moderator 68,426 PointsUsing is
and ==
or !=
are similar but different.
For the three cases you listed, the second one ("A not None") is illegal syntax. Here are three legal syntaxes:
>>> A is not None
True
>>> not A is None
True
>>> A != None
True
The keyword is
relates to two objects being the exact same object, whereas ==
and !=
deal with two objects being equivalent but not necessarily the same object. All objects in Python have an ID related to their location in memory. Two objects are the exact same if they have the same ID:
>>> B = None
>>> C = None
>>> B is C
True
>>> id(B)
9999216
>>> id(C)
9999216
>>> id(None)
9999216
Two object can be equivalent but not the same object:
>>> B = [None]
>>> C = [None]
>>> B == C
True
>>> B is C
False
>>> id(B)
140030159982344
>>> id(C)
140030159808520

Camille Ferré
3,330 PointsHi all,
I have an additional question related to this one.
Why would we write something like:
if books and if books is not None:
books
would be True
if it is indeed not None
right ?
[MOD: added ```python formatting -cf]
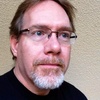
Chris Freeman
Treehouse Moderator 68,426 PointsIn short, it's redundant.
There is a syntax error in your question. The second if
should be removed:
if books and books is not None:
When two conditions are joined with and
, the second condition is only evaluated if the first condition is True
. The first condition is True
if and only if
books
has a "truthy" value. That is, it can't be None
. So if the first condition is True
then the second condition must also be True
. Therefore it is redundant and can be removed leaving:
if books:

Camille Ferré
3,330 PointsThank you Chris, that's what I thought :)
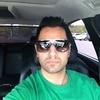
Bikram Mann
Courses Plus Student 12,647 PointsSo, does that mean each value has memory address. and if we assign any variable to that same value it is a pointer to that address ?
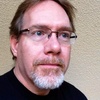
Chris Freeman
Treehouse Moderator 68,426 PointsSometimes, yes, Python may reuse memory addresses for some literal values since they're immutable (can not be altered). But it is extremely rare to write code to that takes advantage of this, so I would not focus on this.
Each object has a location at a specific address. In most cases, python will create a new object with a new address before assigning it to a variable.
$ python3
Python 3.4.3 (default, Oct 14 2015, 20:28:29)
[GCC 4.8.4] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> # reference a string
... "some string"
'some string'
>>> # check id
... id("some string")
140351042828720
>>> # set variable to string
... s = "some string"
>>> # check s id
... id(s)
140351042828720
>>> # python reused existing string
... # reference string with same value
... "some string"
'some string'
>>> # check it's ID
... id("some string")
140351042828784
>>> # Since the first was assigned to s, a new one was created
... # assign same value to s
... s = "some string"
>>> # check s id
... id(s)
140351042828784
>>> # S was changed to point at new copy. Old copy destroyed
... id("some string")
140351042828720
>>> t = "some string"
>>> id(t)
140351042828720
>>> # can not tell if string was the same or if just address was reused.
Some small integers (0-256) are reused, but larger numbers are not:
>>> id(256)
10113984
>>> c = 256
>>> d = 256
>>> c is d is 256
True
>>> id(257)
140351043411856
>>> c = 257
>>> d = 257
>>> c is d is 257
False
>>> id(c)
140351043411856
>>> id(d)
140351042783152
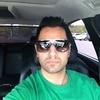
Bikram Mann
Courses Plus Student 12,647 PointsThank for taking the time to explain Chris !! Your time and knowledge is much appreciated :)