Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial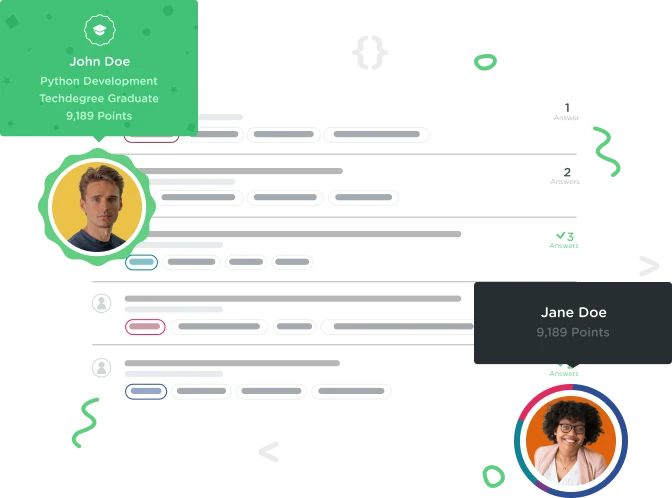
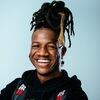
Melvin Mescudi
2,066 PointsDifficulty looping input string prompt to repeat until I get the appropriate value
Trying to get my code to keep retrying until I get a valid input
# Age 18+ Check year to confirm age
while True:
try:
age = int(input("\nWhat year were you born? "))
except ValueError or NameError:
print("*Please enter 4 digit format. Try again")
if age >= int(2004):
print("Sorry, but you don't seem to meet the age requirements :( Please try again.. \n")
if age <= int(2004):
print("\nWelcome oh wise one!...")
break
1 Answer
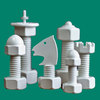
Steven Parker
231,007 PointsHere's a few hints:
- you can't combine error types with logic ("or"), you'll need two "except" statements
- everything involving "age" should be done inside the "try" block
- the inequality test that results in "sorry...." should probably be a "less than" instead of "greater"
- once you change the comparison, you won't need a separate test for 0
- literal digits are already numbers and don't need explicit "int" conversions
Sekhar Gopisetty
1,938 PointsSekhar Gopisetty
1,938 PointsThe issue I see is that you are attempting to convert the user-input to int - which can throw an exception and will cause the loop to end.
I've changed it a bit, and using
isnumeric()
to test if the conversion will succeed or not.