Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial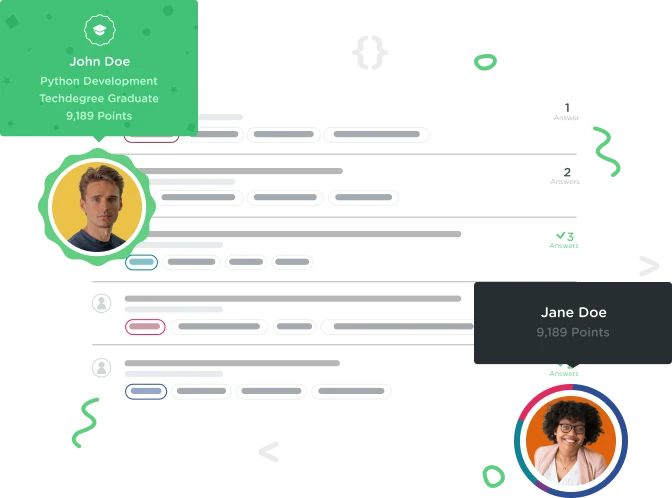
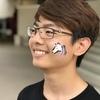
Matt Song
2,971 PointsDon't know why this doesn't work.
I did what I thought should work, but now I'm getting tons of errors and the code "Forum forum = new Forum("Java");" Which I didn't even code, doesn't work also. Someone please help me!
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public void setTopic(String topic) {
topic = "";
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public void User(String firstName, String lastName) {
// TODO: set and add the private fields
firstName = "";
lastName = "";
}
// TODO: add getters for firstName and lastName
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
public void User(User author, String title, String description) {
author = "";
title = "";
description = "";
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User(String getFirstName, String getLastName);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(getAuthor, getTitle, getDescription);
forum.addPost(post);
}
}

Kelvin Warui
Courses Plus Student 878 PointsIn your forum class you seem to be missing a constructor which should have a string argument .You are invoking the default constructor when your create an instance of forum and the error is caused when you try and pass the "java " string to it as the default constructor has no parameter .
1 Answer

Joseph Wasden
20,406 PointsSee the replies from other students for more info. :)
Brian Pedigo
26,783 PointsBrian Pedigo
26,783 PointsHi Matt after quickly looking over your code it seems that in your Forum class you made a setter method instead of constructor
Also your ForumPost class's constructor was off:
Recheck your code for proper constructor creation. Remember constructors do not have a return type. There might be a few more errors but this should get you going in the right direction.