Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial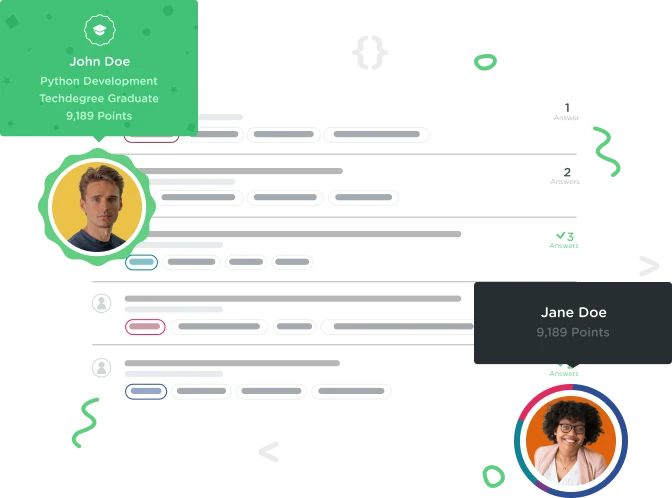
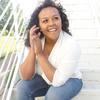
Enzie Riddle
Front End Web Development Techdegree Graduate 19,278 PointsDouble Unders
Watching this video Understanding Dunder Main (main): Double Unders, Ken was saying that when a script is run, Python sets __name__
to __main__
. He said that we can use this to our advantage to check if our script is being run verses being imported. What is the difference between our code being run and our code being imported?
2 Answers

Steven Tagawa
Python Development Techdegree Graduate 14,438 PointsI think the problem is that when this video for double unders and if __name__ == '__main__'
comes up, they haven't really gotten to the concept of importing files yet.
So, you can have different pieces of code in completely different files, and import one file into another. That way you can re-use functions and so on over and over. You could write something like this:
# Here's a function.
def say_hi():
# Do some stuff here...
return "Hi!"
# Here are some commands.
greeting = say_hi()
print("I'm in the hi.py file, and I'm saying: ", greeting)
...and save it in a file called hi.py. (You can even do this in Workspaces.) Then, if you want to use that function in a totally different program, you can write a script in another file, like this:
import hi
aloha = hi.say_hi()
print("Hawaiians say aloha when they mean ", aloha)
...and save it as aloha.py. Now you can use the function from one file in a program in another file. But, now if you run aloha.py, the first thing it does is import the file hi.py, and every bit of code in it will immediately and automatically run. And you'll get this (even if you don't want it):
>>python aloha.py
I'm in the hi.py file, and I'm saying: Hi!
Hawaiians say aloha when they mean Hi!
>>
The only way to stop that from happening is to check to see what the file's __name__
equals. The file that you command to run is always named __main__
, and any file that's imported is given its own filename as a name. So when aloha.py imports the file hi.py, the code inside that file is set to '__hi__'
, not '__main__'
. Since it's been imported and not told to run itself, if __name__ == '__main__'
will be False
, and if you put the code you don't want to always run inside an if __name__ == '__main__'
block, the code won't run unless/until some line in aloha.py specifically tells it to.
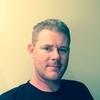
Trevor J
Python Web Development Techdegree Student 2,107 PointsIf you run the follwong code everything below __name__ == '__main__':
will be executed and everything above when you import the code.
# stuff to run always here such as class/def
def main():
pass
if __name__ == '__main__':
# stuff only to run when not called via 'import' here
main()