Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial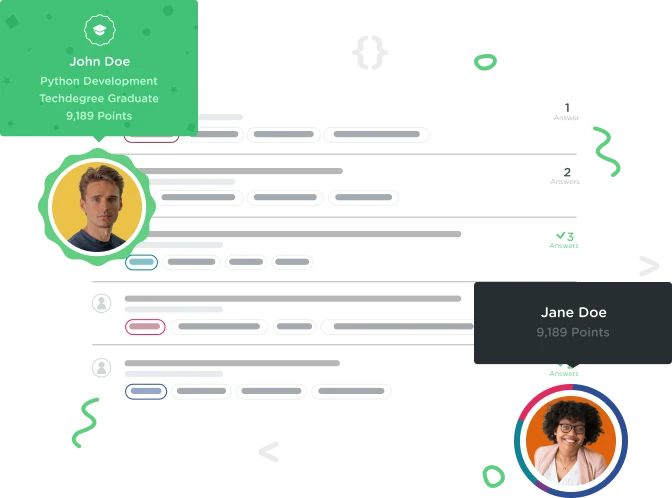

Calvin Lowe
1,309 PointsError for viewing a single article.
I'm having troubles completing the first challenge of creating a new view. Can someone help if I am doing it right? This is the prompt: We need to be able to view a single article. In articles/views.py, create a view named article_detail that takes a pk. The view should return the "articles/article_detail.html" template. Assign the Article to the article key in the context dictionary.
from django.shortcuts import render
from .models import Article, Writer
def article_list(request):
articles = Article.objects.all()
return render(request, 'articles/article_list.html', {'articles': articles})
def writer_detail(request, pk):
writer = Writer.objects.get(pk=pk)
return render(request, 'articles/writer_detail.html', {'writer': writer})
def article_detail(request,pk):
return render(request, "articles/article_detail.html",{'article':Article})
from django.conf.urls import url
from . import views
urlpatterns = [
url(r'writer/(?P<pk>\d+)/$', views.writer_detail),
url(r'', views.article_list),
]
2 Answers

Ryan S
27,276 PointsHi Calvin,
When creating a detail view, you will need to get the object that was requested. Right now you are trying to render an article_detail template, but you don't have the article that belongs to the requested pk. Once you get the article, then you can pass it to the template via the context dictionary.
Here is a hint:
article_detail should be very similar to the writer_detail view.
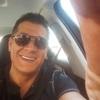
keith rezendes
4,459 PointsThanks, Ryan works.