Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial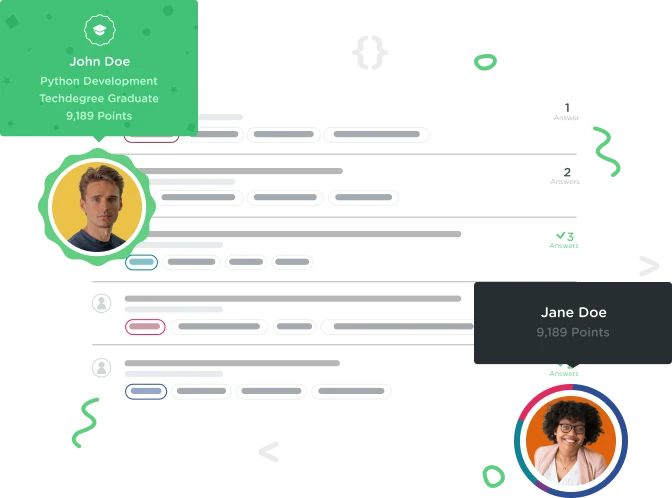
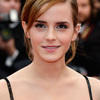
mohamadreza azadi
5,167 Pointsexercise
i'm stuck here and so confused :\ guys please help
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter){
for( char letter:tiles.toCharArray()){
if(tiles.indexOf(letter)!=-1
}
return 1;
}
}
2 Answers
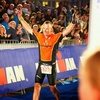
Steve Hunter
57,712 PointsHi there,
You need to create a method called getCountOfLetter
that takes a char
as a parameter and then returns an int
. You've done this already - good work.
Next, you want to iterate through each character in the class member variable tiles
. At each iteration you want to increment a counter variable (I called mine count
which I declared an initialized in the method) if
the char
passed in as a parameter equals the current element of the array.
You've set up the for
loop just fine. However, your loop character is called letter
as is the parameter being passed in - you need to change that; let's follow Thomas' lead and call it l
even if that lacks descriptiveness! Inside you can either check if your current character in the loop (l
) is equal to the character passed in as a parameter to the method (letter
) using an if
statement, which is long handed. If the two equal, increment the count variable. The method returns an integer that represents the number of the parameter character are in the tiles
string. So, If your string is "H E L L O
" and you call getCountOfLetter('L')
, you'll get a 2 returned; the number of L
s in the word HELLO.
So, compare your loop variable l
with letter
using double-equals. If
they are equal, increment the counter - else, move along and do the next letter. Once you have finished looping, return the value of the counter.
That all looks like:
public int getCountOfLetter(char letter){
int count = 0;
for(char l : tiles.toCharArray()){ // [edit - thanks Thomas!]
if(l == letter){
count++;
}
}
return count;
}
However, we've seen how to implement the ternary operator to replace the if
statement, so you can use that instead. It isn't as clear at a quick glance but it is a way of reducing the (tiny) overhead of the if
statement. Thomas showed you how to use : ?
in his answer. Give that a go too!
I hope that helps - let me know how you get on.
Steve.

Thomas Nilsen
14,957 PointsYou weren't too far off;
public int getCountOfLetter(char letter){
//Keep track of count in variable
int count = 0;
//Loop through each letter in string
for(char l : tiles.toCharArray()) {
//If the letter matches the letter we search for, we increment by one
count += (l == letter) ? 1 : 0;
}
//Return the count
return count;
}
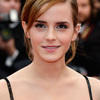
mohamadreza azadi
5,167 Pointsthanks alot
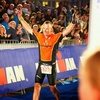
Steve Hunter
57,712 PointsSlick use of the ternary operator; like it!
mohamadreza azadi
5,167 Pointsmohamadreza azadi
5,167 Pointsthanks steve
for complete explanation
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHey, no worries! I hope it made sense and it helped you understand the challenge's solution(s).
Shout if you need anything more.
Steve.
Thomas Nilsen
14,957 PointsThomas Nilsen
14,957 Pointsyou have l == letter, without declaring l anywhere.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsSorry - I amended my code from
letter
tol
and missed that!! Oops! I shall amend it now. Apologies!Done - code-comment added.