Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial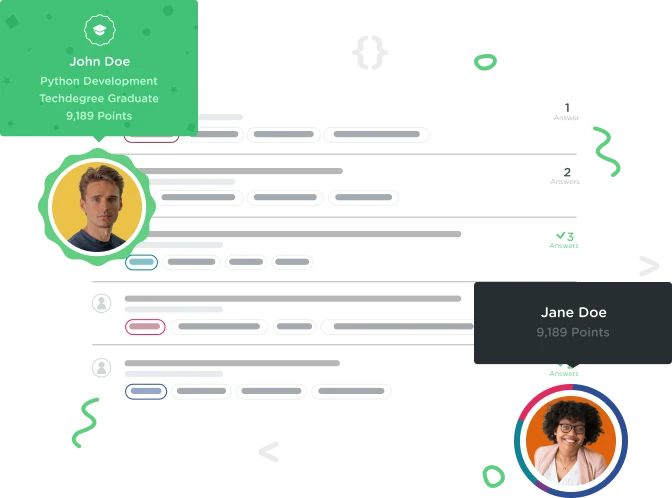

Suraj Shah
1,055 PointsExtending a class
I want my class D20 to extend class Die.
Please could you explain why this does not work:
class D20(Die):
def __init__(self, sides):
super().__init__(sides = 20)
How is it different from this (which works):
class D20(Die):
def __init__(self, sides = 20):
super().__init__(sides)
Thanks!
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self, sides):
super().__init__(sides = 20)
class Hand(list):
@property
def total(self):
return sum(self)
3 Answers
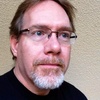
Chris Freeman
Treehouse Moderator 68,441 PointsThe key is in the challenge text "It should automatically have 20 sides and shouldn't require any arguments to create.
# your first example says
class D20(Die):
# __init__ takes one positional argument 'sides'
def __init__(self, sides):
# regardless of argument 'sides',
# call Die.__init__ with keyword argument set to 20
super().__init__(sides = 20) # Note1
# the positional argument 'sides' is not used
# Note1: here, the keyword 'sides' is only related to the parameter "sides" in the Die.__init__ parameter list. It has no relation to any local variable that might also be called "sides"
# your second example says:
class D20(Die):
# __init__ takes one positional argument 'sides'
# with default value 20
def __init__(self, sides = 20):
# call Die.__init__ with positional argument set to argument value or the default 20
super().__init__(sides)
Since the challenge checker doesn't provide the argument 'sides', the first one fails due to missing positional argument. The second one passes only because a default value is defined. The better answer removes sides
parameter.
class D20(Die):
# __init__ takes no positional arguments
def __init__(self):
# call Die.__init__ with keyword argument set to 20
super().__init__(sides = 20)
Post back if you need more help. Good luck!!!

Suraj Shah
1,055 PointsThanks very much Chris Freeman

Ngonidzaishe Tukuta
4,274 PointsThanks Chris Freeman
Igor Ević
5,827 PointsIgor Ević
5,827 PointsIf you use only sides when you create class Die:
you don't need sides in subclass init, because Die20 is special Die with sides = 20:
When you define subclass D20 with:
you must provide sides when you create class Dice20
This works, but only if you create class with Die20() or Die20(20):
What if you call Die20(6)?