Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial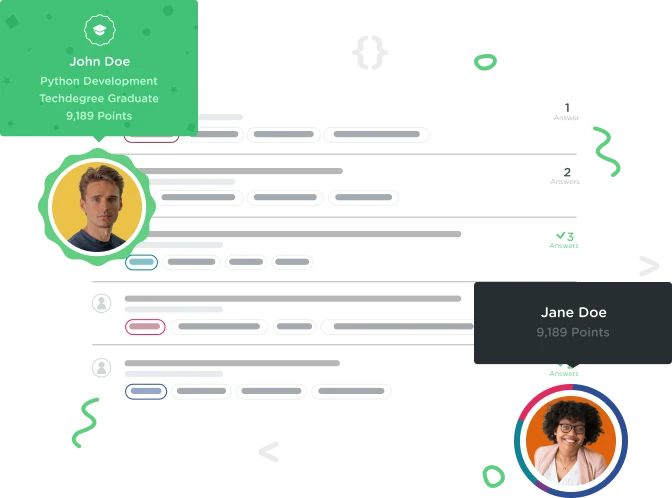
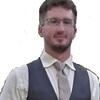
Sean Paulson
6,669 Pointsextra credit: loading static files. now node will not load remote urls from inline code in html/css files
I got my server to load the css file and display on the website. However the @import(font style urls) and <img> tag in the css and html files will not load. In the developer console under the network tab, I get a 400 (bad request) code.
Do I need to code my server to handle png and other urls? If so how come the png and css font imports worked before when the css was in the html header?
here is my code
<img src="http://i.imgur.com/VKKm0pn.png" alt="Magnifying Glass" id="searchIcon">//THIS WONT LOAD SAME WITH THE URLS IN MY CSS FOLDER
<form action="/" method="POST">
<input type="text" placeholder="Enter a Treehouse username" id="username" name="username">
<input type="submit" value="search" class="button">
</form>
const router = require('./router.js');
//problem: Need a simple way to look at a users badge count and JavaScript point from a web browser
//solutions: Use node.js to perform profile look ups and serve our template via HTTP
//1. Create web server
const http = require('http');
const https = require('https');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
let username = req.url.replace("/", "");
console.log('******searching for server req******');
if(req.url.indexOf('.css') !== -1) {
router.style(req, res);
}
if(req.url === "/") {
router.home(req, res);
}
if(username.length > 0 && username !== 'recources/index.css') {
router.user(req, res);
}
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
let Profile = require("./profile.js");
const renderer = require('./renderer.js');
const querystring = require('querystring');
//Handle HTTP route GET / and POST / i.e. Home
function style(req, res) {
res.setHeader('Content-Type', 'text/css');
console.log('******inside style******');
renderer.css('index', res);
res.end();
}
function home(req, res) {
console.log('******Calling Home******');
if(req.method.toLowerCase() === "get") {
console.log(req.url);
console.log('******Calling get******');
res.setHeader('Content-Type', 'text/html');
renderer.view('header', {}, res);
renderer.view("search", {}, res);
renderer.view("footer", {}, res);
res.end();
//if url === "/" POST
}else {
console.log('******POSTing home func******');
//get the post data from body
//extract username
//redirect to /:username
req.on('data', (postBody) => {
let query = querystring.parse(postBody.toString());
res.writeHead(303, {"Location": "/" + query.username });
res.end();
});
}
}
//Handle HTTP route GET /username i.e. /chalkers
function user(req, res) {
let username = req.url.replace("/", "");
console.log('*****calling user***'+ username +'***');
console.log('*******in user*****');
res.setHeader('Content-Type', 'text/html');
renderer.view("header", {}, res);
let studentProfile = new Profile(username);
studentProfile.on("end", (profileJSON) => {
let values = {
avatarUrl: profileJSON.gravatar_url,
badges: profileJSON.badges.length,
username: profileJSON.profile_name,
javascriptPoints: profileJSON.points.JavaScript
}
//simple response
renderer.view("profile", values, res);
renderer.view("footer", {}, res);
res.end();
});
studentProfile.on("error", (error) => {
//show Error
renderer.view('error', {errorMessage: error.message}, res);
renderer.view("search", {}, res);
renderer.view("footer", {}, res);
res.end();
});
}
module.exports.style = style;
module.exports.home = home;
module.exports.user = user;
const fs = require('fs');
function mergeValues(values, content) {
//cycle over the keys
for(let key in values) {
//replace{{key}} with the value from the values param
content = content.replace("{{" + key + "}}", values[key]);
}
return content;
//return merged content
}
function view(templateName, values, res) {
//Read from the template file
console.log('******rendering Data******');
let data = fs.readFileSync(`./views/${templateName}.html`, 'utf8');
//insert values into the content
data = mergeValues(values, data)
//write out to the response
res.write(data);
}
function css(fileName, res) {
let data = fs.readFileSync(`./views/recources/${fileName}.css`, "utf8");
res.write(data);
}
module.exports.view = view;
module.exports.css = css;
4 Answers
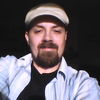
Robert Manolis
Treehouse Guest TeacherHi Sean Paulson, I'm not sure why that isn't working, but I'd like to take a closer look. Can I talk you into creating a GitHub repo, adding these project files to it and then sharing a link here so I can run the code myself and test it out, pretty please? I'll let you know if I find anything.
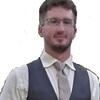
Sean Paulson
6,669 PointsLooks like I can get Other Images to load from a different url. I guess my request to imgur is being blocked by a bad request or CORS or something.
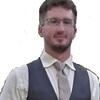
Sean Paulson
6,669 PointsAwesome here is the GitHub repo https://github.com/SeanPaulson/NodeJS-Server-Practice Thank you for the help. This one has really stumped me. When I google I see other ways I can do this. mainly using express. Only in the spirit of learning I would like to know. :)
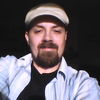
Robert Manolis
Treehouse Guest TeacherAhh, okay, I see what's going on here now, Sean Paulson. I didn't realize at first that this was all built with raw Node.js. Typically you would use the super handy Express web app framework, which is a big help with serving up those static files. Here's a great Treehouse community page with some info that I think will be helpful.
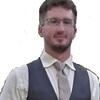
Sean Paulson
6,669 PointsHey thanks! Robert Manolis that link did clear some things up. Yes this i am trying to use raw nodejs and not express.
Although I wanted to clarify, I can get the CSS to load but the inline CSS @import(url google fonts) links and inline HTML<img> tags with remote URLs will not load. The html should load a https://i.imgur.com/VKKm0pn.png img when you go to the home page. This file is inline in the html
<img src="http://i.imgur.com/VKKm0pn.png" alt="Magnifying Glass" id="searchIcon">
Did This image load for you on the home screen? (I get a 403 error code)
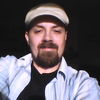
Robert Manolis
Treehouse Guest TeacherHey Sean Paulson, yeah the magnifying glass image showed up fine for me when I ran the node app
command and fired up the app. If I'm remembering correctly, the font imports were an issue. As was the CSS file when I tried to hook that up to the CSS. These connections get trickier on the server side. But Express can be a big help with things like that.