Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial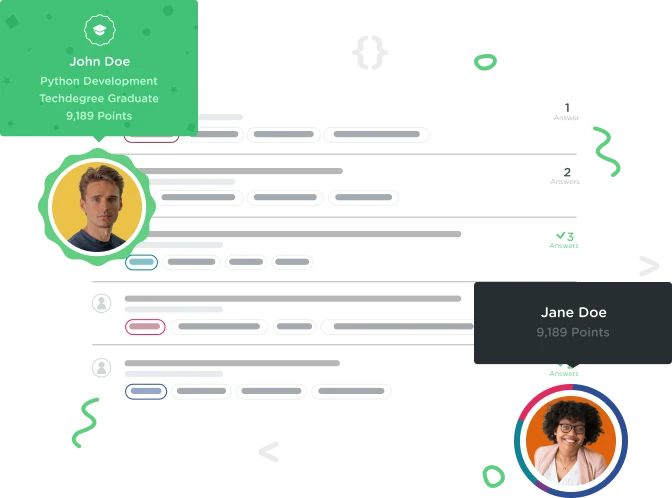

rob bert
5,041 PointsFeeling like im missing something obvious in this code.
So, i feel like this should work, but it doesnt, i also feel like im missing something painfully obvious.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
out = ''
for char in self.pattern:
if char == '.':
out += dot
elif char == '-':
out += 'dash'
out += '-'
out = out[:-1]
return out
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer

andren
28,558 PointsThere are two issues in your code:
- In this line
out += dot
you have forgotten to wrap the word dot in quotes. - The challenge specifies that dashes are represented by an
_
symbol (that's an underscore, not a dash).
If you fix those two issues like this:
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
out = ''
for char in self.pattern:
if char == '.':
out += "dot" # Wrap dot in quotes
elif char == '_': # Check if `char` is an underscore rather than a dash
out += 'dash'
out += '-'
out = out[:-1]
return out
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
Then your code will pass.