Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial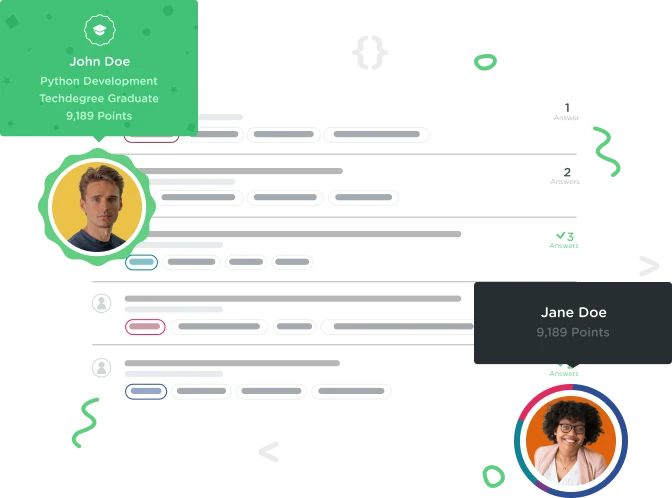
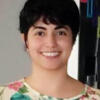
Ingrid Oncken
216 PointsFizzBuzz Challenge, I can't make it work.
I have written and rewritten it so many times, I have also read somewhere here that it's not necessary to write down the condition | is_fizz == 0 and is_buzz != 0:|
but I also don't understand why. To finish my problems I can't find the resolution video 😳.
# TODO: Print out the User's name and the number entered,
# making sure the two statements are on separate lines of output.
#asking for the gamer name
name = input("Please enter your name: ")
#asking for the number
number = int(input("Please enter a number: "))
#QUESTION: Is there a way to show a message to the user in case he/she enter a letter instead of a number?
# TODO: Define variables for is_fizz and is_buzz that stores a Boolean value of the condition. Remember that the modulo operator, %, can be used to check if there is a remainder.
# TODO: Compare the number the user gave with the different
is_fizz = (number % 3 == 0)
is_buzz = (number % 5 == 0)
is_fizzbuzz = (is_fizz == 0 and is_buzz == 0)
# If "is a Fizz number."
if is_fizz == 0 and is_buzz != 0:
print("{} is a Fizz number!".format(number))
# If "is a Buzz number."
elif is_buzz == 0 and is_fizz != 0:
print("{} is a Buzz number!".format(number))
# FizzBuzz conditions.
elif is_fizzbuzz == 0:
print("{} is a FizzBuzz number!".format(number))
#"is neither a fizzy or a buzzy number."
else:
print("{} is neither a fizzy or a buzzy number.".format(number))
# *********************
Thank you for your answer(s)!
1 Answer
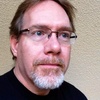
Chris Freeman
Treehouse Moderator 68,441 PointsThere seem to be some polarity reversals. Treating the False/True
values as 0/non-0
is valid since zero is a "falsey" value and non-zero numbers are considered "truthy".
Put into a logical context, the result of these comparisons result in a True
or False
value:
is_fizz = (number % 3 == 0)
is_buzz = (number % 5 == 0)
is_fizzbuzz = (is_fizz == 0 and is_buzz == 0)
One simplification would to simplify the later comparisons. Instead of comparing a Boolean value to 0, the value can be reference directly:
# use:
if not is_fizz:
# in place of
if is_fizz == 0:
Rewriting the first comparisons:
# If "is a Fizz number."
if is_fizz == 0 and is_buzz != 0:
# becomes
if not is_fizz and is_buzz: # 'is_buzz' same as 'not not is_buzz'
Using this rewrite it becomes clearer that this code would be better for determining a Buzz..
Sometime you can reduce the overall logic knowing that the order you check some states eliminates the need for checking all conditions for other states. For example,
# check if both is_fizz and is_buzz => must be FizzBuzz
# check if is_fizz => must be Fizz
# check if is_buzz ==> must be Buzz
Post back if you need more help. Good luck!
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Ingrid,
Haven't really dug into your code, but I think the issue is that you're testing if is_fizz/is_buzz are equal to 0. But the variables you've created is_fizz/is_buzz hold boolean values (True/False).
So essentially you're testing stuff like if True == 0, which gives you false. Remove the comparison operators from your conditional statements (i.e.
if is_fizz and is_buzz: print("{} is a Fizz number!".format(number))
) and I think you'll get better results.