Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial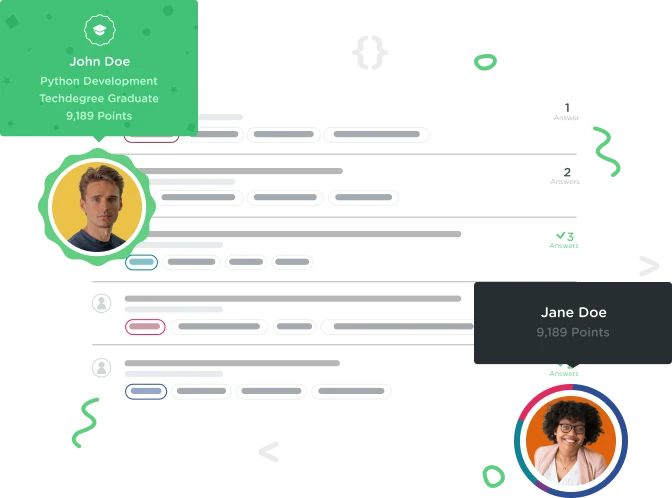

Matt Otis
1,402 PointsFrustration.py and Super() Value Mart
Seems like this code should work....
class Liar(list):
def __len__(self):
actual_len = super().__len__(self)
dummy_len = 5
if actual_len == dummy_len:
dummy_len += 1
return dummy_len
But, I’m getting return of...
TypeError: expected 0 arguments, got 1
Why would it expect 0 arguments when I’m clearly trying to call a method and get a value?
Also, where am I going wrong?
2 Answers

Anthony Crespo
Python Web Development Techdegree Student 12,973 PointsThe function __ len__ actually don't take any argument.
If you take the argument off your code it will work.
class Liar(list): def __len__(self): actual_len = super().__len__(). # don't need to pass anything here dummy_len = 5 if actual_len == dummy_len: dummy_len += 1 return dummy_len
I just started learning python but this is how I understand it.
Normally, when you want to call the len() function in your code you will do this:
a = [1,2,3] len(a)It tells to the variable "a" to call the function __ len__ and it will return the length of it. It mean you could do:
a = [1,2,3] a.__len__()
Like you can see len() who is __ len__() don't need a variable it already knows you want to return the length of "self".
class Liar(list):
def __len__(self): # this function override the __len__ function of list...
actual_len = super().__len__(). # ...but you can still use it if you want and get the length like before you override it
dummy_len = 5
if actual_len == dummy_len:
dummy_len += 1
return dummy_len
Use self.function_name to call a function in your class and super().function_name to call one of the function your class inherited.

Matt Otis
1,402 PointsThanks for your insight. I hadn’t quite put together that:
len(a) == a.__len__()
That and your insight about self.function_name
and super().function_name
was super helpful in helping me ‘get’ it.
Thanks Anthony!
fwiw this is the code that passed:
class Liar(list):
def __len__(self):
actual_len = super().__len__()
dummy_len = 5
if actual_len == dummy_len:
dummy_len += 1
return dummy_len

Anthony Crespo
Python Web Development Techdegree Student 12,973 PointsNo problem I’m glad I could help! I had the same question when I did that part of the course.