Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial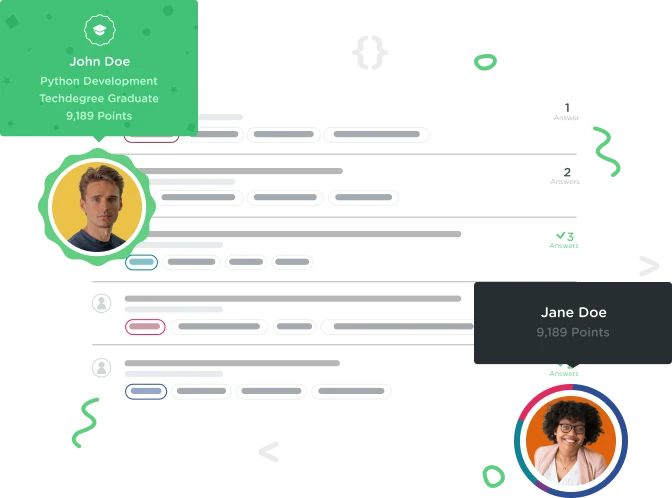
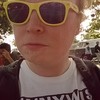
Jeremy O'Bryan
16,672 PointsFunction to add items to a database in Flask
Hi everyone!
I'm working on a Flask app that will be running on my local web server; essentially it will be a sort of "personal dashboard" that will have things like notes, a to-do list, a shopping list, etc. So far I have been able to get the 'to-do list' part working by setting up the 'Todo' database like so:
class Todo(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100))
complete = db.Column(db.Boolean)
and then creating the 'add item' function:
def add():
#add new item
title = request.form.get("title")
new_todo = Todo(title=title, complete=False)
db.session.add(new_todo)
db.session.commit()
return redirect(url_for("index"))
Where I'm struggling is figuring out how to re-use that function for the other dbs that have been created (like the shopping list db). I could probably create a different function to add / remove items for each db but I feel like that wouldn't be very Pythonic :D , any suggestions would be incredibly helpful!
3 Answers
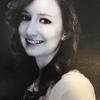
Megan Amendola
Treehouse TeacherI would suggest making the add function @classmethod for the model classes.
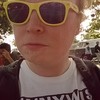
Jeremy O'Bryan
16,672 PointsThanks Megan! I think I'm following so far, so would I do something like this?
class Dbase(self, name):
dbname = name(db.Model)
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100))
complete = db.Column(db.Boolean)
@classmethod
def add():
#add new item
title = request.form.get("title")
new_item = dbname(title=title, complete=False)
db.session.add(new_item)
db.session.commit()
What I'm thinking this will do is create a class for a database and a class method for add, so I can then re-use the 'Dbase' class to create a new database name which I will define, and then I can re-use the add function for the db that I created with that class?
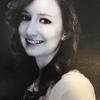
Megan Amendola
Treehouse TeacherI would give each DB model class its own @classmethod
since each model is most likely going to be unique.
class Todo(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100))
complete = db.Column(db.Boolean)
@classmethod
def add(cls, title, complete):
new_todo = cls(title=title, complete=complete)
db.session.add(new_todo)
db.session.commit()
class Cars(db.Model):
id = db.Column(db.Integer, primary_key=True)
make = db.Column(db.String(100))
model = db.Column(db.String(100))
@classmethod
def add(cls, make, model):
new_car = cls(make=make, model=model)
db.session.add(new_car)
db.session.commit()
Then you would call Todo.add('some title', False)
and Car.add('ford', 'mustang')
to add items to each database.
You could create something like this as well to create only one function.
def add_to_db(Class, **kwargs):
new_instance = Class(**kwargs)
db.session.add(new_instace)
db.session.commit()
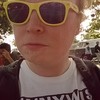
Jeremy O'Bryan
16,672 Pointsawesome I get it, thanks!