Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial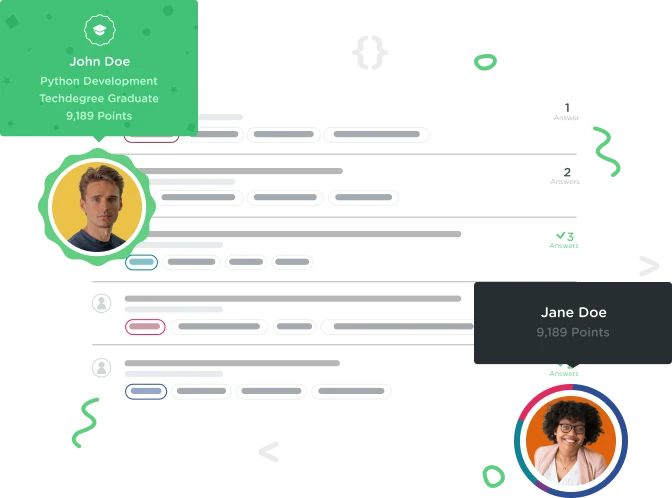
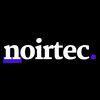
emmanuel egunjobi
4,475 PointsGetting a 'Uncaught SyntaxError: Identifier 'astrosUrl' has already been declared
I don't know why I am getting this error as I don't see the variable being change within functions
/// Getting a 'Uncaught SyntaxError: Identifier 'astrosUrl' has already been declared
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
function getJSON(url) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => {
if(xhr.status === 200) {
let data = JSON.parse(xhr.responseText);
resolve(data);
} else {
reject( Error(xhr.statusText) );
}
};
xhr.onerror = () => reject( Error('A network error occured.') );
xhr.send();
})
}
function getProfiles(json) {
const profiles = json.people.map( person => {
return getJSON(wikiUrl + person.name);
});
return profiles;
}
// Generate the markup for each profile
function generateHTML(data) {
const section = document.createElement('section');
peopleList.appendChild(section);
// Check if request returns a 'standard' page from Wiki
if (data.type === 'standard') {
section.innerHTML = `
<img src=${data.thumbnail.source}>
<h2>${data.title}</h2>
<p>${data.description}</p>
<p>${data.extract}</p>
`;
} else {
section.innerHTML = `
<img src="img/profile.jpg" alt="ocean clouds seen from space">
<h2>${data.title}</h2>
<p>Results unavailable for ${data.title}</p>
${data.extract_html}
`;
}
}
btn.addEventListener('click', (event) => {
getJSON(astrosUrl)
.then(getProfiles)
.then( data => console.log(data) )
.catch(err => console.log(err));
event.target.remove();
});
2 Answers
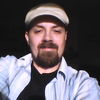
Robert Manolis
Treehouse Guest TeacherHey emmanuel egunjobi , I just copied your code and ran it myself, and I'm not seeing that error. Perhaps you have that variable defined in another JS file that's hooked up to the same index.html file. In client side JS, all the JS files that are wired up to the HTML share the same global scope. So if you define a variable with const in one of those files, and then try to define the same variable in another file, you'll get an error like that.
After I ran the above code, I got an array of promises printing to the console. Once I change the final return statement of the getProfiles function to return Promise.all(profiles);
, then I got an array of objects containing astronaut info instead.
Hope that helps!
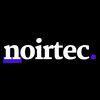
emmanuel egunjobi
4,475 PointsHi Robert Manolis Thank you for your help I just took another look at the code and see that I have two script tags and one is pointing to another js file which has same variable. I need better glasses lol
Thanks again