Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial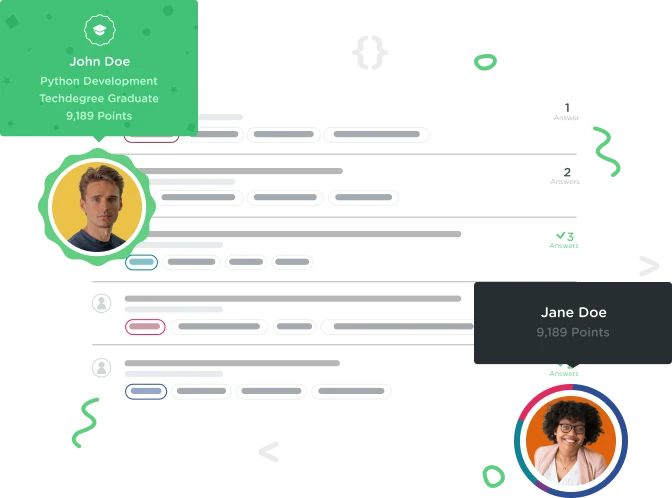

Lindsey Mote
Data Analysis Techdegree Student 1,148 PointsGetting Closer on Guessing Game - Help with ValueError and Restart
I've made significant progress on the number guessing game but still have a few issues:
I'm making a mistake with the ValueError code but can't figure out where that error is so it's hashed out for now.
The game allows 5 guesses but still asks for another guess before printing the "out of guesses..."
The game ends appropriately if I say I do not want to play again, but does nothing if I say yes. Not clear how to restart it.
I appreciate any help I get. I've actually been trying to hire a tutor for two weeks but three have flaked out of me so I'm left with hours of trial and error - which is fine but frustrating.
#def start_game():
import time
import random
from statistics import mode, median, mean
game_solution = random.randint(1, 20)
attempt = 5
print("Welcome to Lindsey's Guessing Game!")
time.sleep(1)
play_game = input("Would you like to play? Yes or No ")
if play_game.lower() == "no":
print("Have a great day!")
if play_game.lower() == "yes":
guesses = []
print("Please choose a number between 1 and 20. ")
# try:
# raise ValueError()
# except ValueError as err:
# print("Please enter a valid number.".format(err))
while attempt > 0:
player_guess = int(input(""))
if player_guess < game_solution:
print("Nope, too low! Guess again: ")
elif player_guess > game_solution:
print("Nope, too high! Guess again: ")
else:
print("You guessed it! Nice job!")
time.sleep(1)
play_again = input("Would you like to play again? Yes or No ")
attempt -= 1
guesses.append(player_guess)
if attempt < 5:
print("Sorry, you are out of guesses. The answer was {}.".format(game_solution))
time.sleep(1)
play_again = input("Would you like to play again? Yes or No ")
while play_again.lower() == "yes":
play_game
if play_again.lower() == "no":
print("You made {} guesses.".format(len(guesses)))
time.sleep(0.5)
print("Your mode guess was {}.".format(round(mode(guesses))))
time.sleep(0.5)
print("Your median guess was {}.".format(round(median(guesses))))
time.sleep(0.5)
print("Your mean guess was {}.".format(round(mean(guesses))))
time.sleep(0.5)
print("Thanks for playing! Have a great day :)")
2 Answers
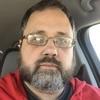
Mark Sebeck
Treehouse Moderator 37,777 PointsHi Lindsey. Good attempt and most of it does work. Couple of issues. First remember DRY. Don't repeat yourself. you check many times if they want to play again. Check only once at the top of a loop. I would do something like:
while 1=1:
play_game = input("Would you like to play? Yes or No ")
if play_game.lower() == "no":
exit
#rest of your code
print("Have a great day!")
I would start that loop right after your welcome. if they want to play your code will run. if not it will exit that loop. so when the game is over that loop will run again and will ask them again. It's an infinite loop that only breaks if they say no.
now your main loop where they guess works but you need a way out if they guess correctly. so if they guess add an exit. and instead of checking if attempts is less than 5 check if it's 0. If it's 0 they didn't guess it. if it's greater than than 0 they did (even if they use 5 guess you haven't subtracted one yet so it will still = 1.
I hope this helps and post back to the community if you are still stuck. best of luck. you can do it!

jb30
44,806 Points try:
raise ValueError()
except ValueError as err:
print("Please enter a valid number.".format(err))
will raise a ValueError and print "Please enter a valid number."
To catch and print the ValueError if the user input cannot be converted to an integer, you could use
try:
player_guess = int(input(""))
except ValueError as err:
print("Please enter a valid number. {}".format(err))
else:
if player_guess < game_solution:
# ...
Currently, if you guess the number correctly, the line play_again = input("Would you like to play again? Yes or No ")
stores the input into play_again
, and that value is not used. While there are attempts left, the user continues the game with game_solution
unchanged and without the number of attempts being refilled.
In the lines
while play_again.lower() == "yes":
play_game
The value of play_again
is not changed by the value of play_game
, so it is an infinite loop. You could break the loop by changing the value of play_again
within the while
block, such as
while play_again.lower() == "yes":
play_again = input("Would you like to play again? Yes or No ")