Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial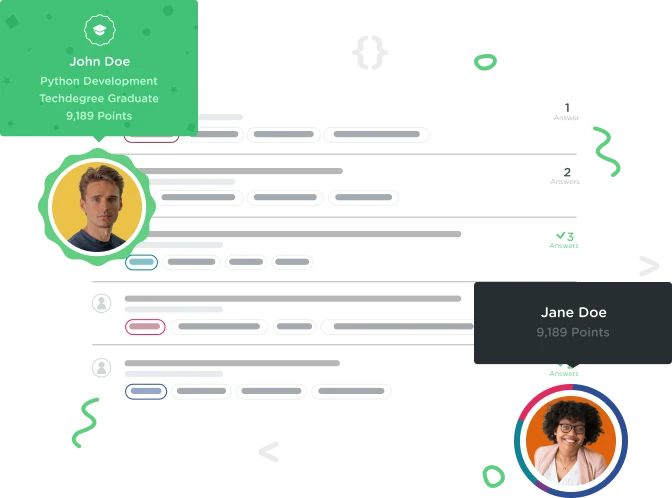

Isak Landström
2,882 PointsHangman game, trouble with open a file.
Hello guys!
I'm working on making a simple hangman game and everything works mostly as expected but I have a trouble when I want to restart the game after you lost/won. This code part is the problem:
retry = input("Do you want to retry? (Y/N) ").lower()
if retry == "y" or retry == "yes":
outer_loop = True
I get the following error:
"Traceback (most recent call last):
File "/home/treehouse/workspace/hangman.py", line 36, in <module>
secret_word = secret_word()
TypeError: 'str' object is not callable "
PS: I am not done with the game and I have still some error handling to take care of but I got stuck on this problem, anyone can help me out?
import random
import hangman_list
def draw_hangman():
for hangman in hangman_s[len(bad_guesses) - 1:len(bad_guesses)]:
print(hangman)
def secret_word():
f = open("hangman_words.txt", "r")
hangman_words = f.read().split()
secret_word = random.choice(hangman_words)
return secret_word
def print_alphabet():
for c in alphabet:
if c not in bad_guesses:
print(c.upper() + " ", end="")
else:
print("_", end=" ")
def draw_secret_word():
print("\n")
for c in secret_word:
if c in good_guesses:
print(c.upper() + " ", end="")
else:
print("_" + " ", end="")
alphabet = "abcdefghijklmnopqrstuvwxyz"
outer_loop = True
game = True
while outer_loop:
secret_word = secret_word()
hangman_s = hangman_list.Hangman.hangman_1
good_guesses = set()
bad_guesses = set()
while game:
print("\nPlease write a single character that you think is in the secret word from the following list >>> ")
print_alphabet()
draw_secret_word()
guess = input()
if guess in secret_word:
print(f"The letter {guess} is in the word!")
good_guesses.add(guess)
draw_secret_word()
if good_guesses == set(secret_word):
print(f"\nYou figured out the secret word: {secret_word}\n Do you want to replay? (Y/N) ")
retry = input("")
# ask if the user wants to retry
elif guess is not secret_word and len(guess) < 2:
bad_guesses.add(guess)
draw_hangman()
print(f" {guess} is not in the word")
if len(bad_guesses) > 7:
print("Sorry you lost!")
break
else:
print("Invalid input, single characters only!")
retry = input("Do you want to retry? (Y/N) ").lower()
if retry == "y" or retry == "yes":
outer_loop = True
else:
print("Okay. thank you for playing!")
outer_loop = False
1 Answer

Isak Landström
2,882 PointsHello again, so I'm kind of answering my own question but not really..
I updated my code so it works now, things look a little smoother, please check it out and if you have some feedback I would love to hear it :)
import random
import hangman_list
import os
def clear_screen():
os.system('cls')
os.system('clear')
def draw_hangman():
for hangman in hangman_s[len(bad_guesses) - 1:len(bad_guesses)]:
print(hangman)
def print_alphabet():
for c in alphabet:
if c not in bad_guesses:
print(c.upper() + " ", end="")
else:
print("_", end=" ")
def draw_secret_word():
print("\n")
for c in secret_word:
if c in good_guesses:
print(c.upper() + " ", end="")
else:
print("_" + " ", end="")
alphabet = "abcdefghijklmnopqrstuvwxyz"
outer_loop = True
game = True
while outer_loop:
with open("hangman_words.txt", "r") as f:
hangman_words = f.read().split()
secret_word = random.choice(hangman_words)
hangman_s = hangman_list.Hangman.hangman_1
good_guesses = set()
bad_guesses = set()
while game:
print("\nPlease write a single character that you think is in the secret word from the following list >>> ")
draw_hangman()
print_alphabet()
draw_secret_word()
guess = input()
clear_screen()
if guess in secret_word:
print(f"The letter {guess} is in the word!")
good_guesses.add(guess)
if good_guesses == set(secret_word):
print(f"\nYou figured out the secret word: {secret_word}\n Do you want to replay? (Y/N) ")
retry = input("")
# ask if the user wants to retry
elif guess is not secret_word and len(guess) < 2:
bad_guesses.add(guess)
clear_screen()
print(f" {guess} is not in the word")
if len(bad_guesses) > 7:
print("Sorry you lost!")
break
else:
print("Invalid input, single characters only!")
retry = input("Do you want to retry? (Y/N) ").lower()
if retry == "y" or retry == "yes":
good_guesses.clear()
bad_guesses.clear()
else:
print("Okay. thank you for playing!")
outer_loop = False