Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial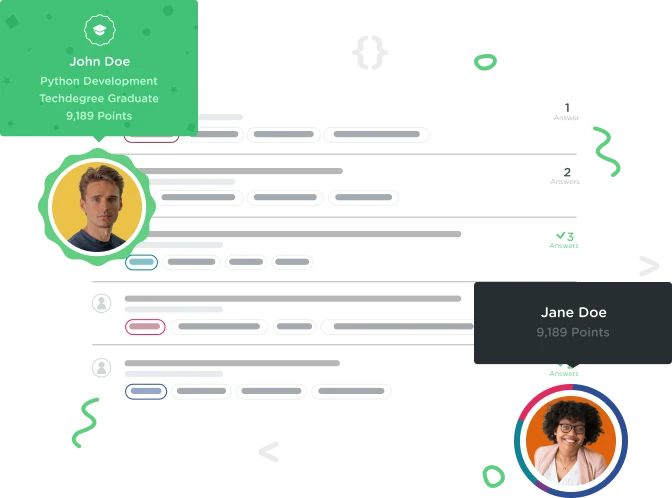

Cármen Fonseca
Courses Plus Student 619 PointsHangman.java:7: error: cannot find symbol Prompter prompter = new Prompter(game);
Keep getting this error. Is it a typo? Thanks
Prompter prompter = new Prompter(game);
^
symbol: class Prompter
location: class Hangman
Hangman.java:8: error: cannot find symbol
Prompter prompter = new Prompter(game);
^
symbol: class Prompter
location: class Hangman
2 errors
My code:
Hangman.java:
public class Hangman {
public static void main(String[] args) { // Your incredible code goes here...
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
boolean isHit = prompter.promptForGuess();
if(isHit){
System.out.println("It's a hit!");
} else {
System.out.println("It's a miss!");
}
} }
Prompter.java:
import java.util.Scanner;
class Prompter { private Game game;
public Prompter(Game game) { this.game = game; }
public boolean promptForGuess(){ Scanner scanner = new Scanner(System.in); System.out.print("Enter a letter: "); String guessInput = scanner.nextLine(); char guess = guessInput.CharAt(0); return game.applyGuess(guess); } }
Game.java:
class Game { private String answer; private String hits; private String misses;
public Game(String answer){ this.answer = answer; hits = ""; misses = ""; }
public boolean applyGuess(char letter){ boolean isHit = answer.indexOf(letter) != -1; if (isHit){ hits += letter; } else { misses += letter; } return isHit; } }
2 Answers

Benjamin Deollos
2,097 PointsSo one of things that I have found. Your syntax for the CharAt should have a lower case "c" in the prompter class. It should look like this :
import java.util.Scanner;
public class Prompter { private Game game;
public Prompter(Game game) { this.game = game; }
public boolean promptForGuess() { Scanner scanner = new Scanner(System.in); System.out.print("Enter a letter: "); String guessInput = scanner.nextLine(); char guess = guessInput.charAt(0); <------// Replace the capital C with a lower case c return game.applyGuess(guess);
} }
Everything else looks good. Try to make those changes and keep us posted. Happy learning

Paolo Bass
3,921 PointsHi Carmen,
I believe the error is due to you not importing or explicitly stating the location of Prompter. I'm not entirely certain of the package structure of this program, however I will try to explain.
If a class is located in another package, you must import or explicitly state the location of the class. If the Prompter class is located in another package, you need to either import the Prompter class by saying:
import (packageName).Prompter;
or:
(packageName).Prompter prompter = new (packageName).Prompter(game);
If the Prompter class is in the same package as the Hangman class, then I'm not entirely sure what the problem is.
I hope that this helped.
Tracy Chacon
7,372 PointsTracy Chacon
7,372 PointsHave you found the answer to this issue?