Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial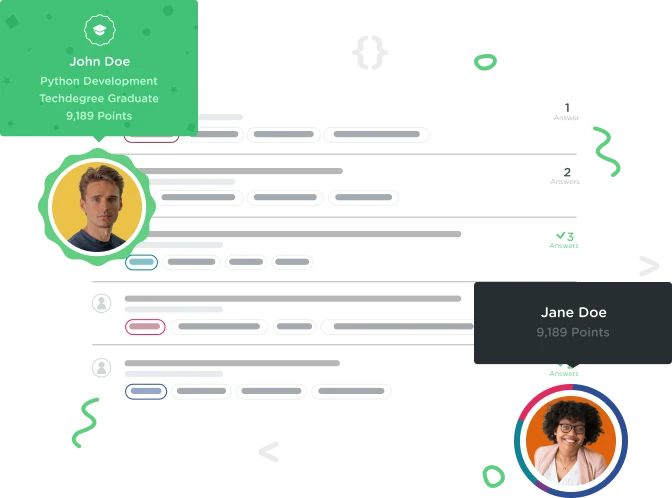

Andrei Oprescu
9,547 PointsHave I done something wrong?
Hi!
I am currently on a challenge on a course but I don't know what I did wrong.
My challenge question is this:
And, finally, if I have doubles, I want to reroll the hand. Add a classmethod to CapitalismHand named reroll that returns a new instance of the class, effectively rerolling the hand.
I have tried multiple times but I just couldn't do it.
My code for this challenge is at the bottom of this question.
Can someone help me?
Thanks!
from dice import D6
class Hand(list):
def __init__(self, size=2, die_class=D6, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
@property
def doubles(self):
if self[0] == self[1]:
return True
return False
@classmethod
def reroll(cls):
if doubles == True:
Hand().__init__()
return cls()
3 Answers
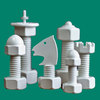
Steven Parker
231,007 PointsThe "reroll" method should always return a new instance. It should not have any conditional logic. And the line that references "Hand" directly isn't needed.
But didn't you create an override method for "__init__
" in task 1? I don't see that here.

grantcenter
8,421 PointsIm still a bit confused on not needing to use a conditional here as well.
why/how would you not need to check if doubles is true if that's the only time you want to do this?
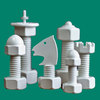
Steven Parker
231,007 PointsThe "reroll" method simply rolls a new hand. The instructions talk about using it after finding doubles, but double detection is done separately (by the "doubles" method).
We also don't know if that's the only time this method would be used.

Andrei Oprescu
9,547 PointsHello,
Can you please explain what you mean by 'conditional logic'? And also I was not supposed to override the 'init'
Thanks!
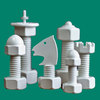
Steven Parker
231,007 PointsAn "if" statement implements conditional logic.
How did you pass task 1 without overriding "__init__
"?
Andrei Oprescu
9,547 PointsAndrei Oprescu
9,547 PointsTask one was only to change the value of size to 2 and change the dice_class to 'D6'
Steven Parker
231,007 PointsSteven Parker
231,007 PointsOh I see what happened, you "fooled" the validation. But the instructions said "finish out the CapitalismHand class", so that's where all the code should be done. They didn't intend for you to modify the "Hand" class.
Andrei Oprescu
9,547 PointsAndrei Oprescu
9,547 PointsSo I have changed the 'init' to its normal and modfied the init in the CapitalismHand.
Now what should I do when I have this code in my class?:
@classmethod def reroll(cls): if doubles == True: return cls()
How am I supposed to complete this without any conditional logic?
Steven Parker
231,007 PointsSteven Parker
231,007 PointsThe new method "returns a new instance of the class". It always does this, so there's no need to test anything.
Andrei Oprescu
9,547 PointsAndrei Oprescu
9,547 PointsOh thanks!
It worked, I thought that when it said "if I had doubles" I had to include a conditional statement.
Thanks!