Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial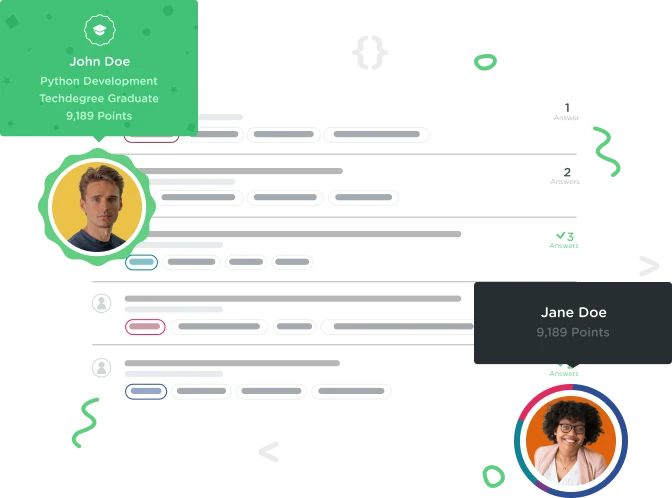

johnpatrick2
3,647 PointsHaving trouble with a test of defining a function
Here is a simple test I am doing to see how defined functions work. I do not understand the indent error I am getting.
Set thermostats to Heat if dining room temperature is less than 60 else set them to Cool
def finish_up():
current_temp = 60
print(current_temp)
if current_temp < 60:
mode = "Heat"
else:
mode = "Cool"
finish_up
print(mode)
print("The function has completed its job")
1 Answer

johnpatrick2
3,647 PointsI am still having trouble with this. The function works. However, I cannot get access to the variables outside of the function. I simplified the script so I could focus on the problem but cannot figure it out. Must be something simple. Here is the script followed by error messge.
def finish_up(temp, mode):
if temp < 60:
mode = "Heat"
else:
mode = "Cool"
print(mode)
print(temp)
return temp, mode
finish_up(99,"Neutral")
print(mode) print(temp) print("The function has completed its job")
treehouse:~/workspace$ python indigo.py
Cool
99
Traceback (most recent call last):
File "indigo.py", line 15, in <module>
print(mode)
NameError: name 'mode' is not defined
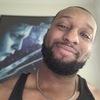
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi johnpatrick2,
The variables within the finish_up function are locally scoped, and so they can't be accessed in the global scope (or a scope that's not a descendant of the scope they're created in).
If you need access to the values, you can return them from the function. Here's an example.
from random import randint
pixar_movie_quotes = [
"To infinity and beyond",
"Adventure is out there",
"He touched the butt",
"Honey, where is my super suit?",
"Just keep swimming"
]
def getRandomQuote(list_of_quotes):
# randint returns a random integer between two values |
# I'm using the number to index into the list of quotes
selected_quote = list_of_quotes[randint(0, len(list_of_quotes) - 1)]
return selected_quote
getRandomQuote(pixar_movie_quotes)
# The try block below will raise an exception, because
# outside of getRandomQuote, I don't have access to the selected_quote variable
try:
print(selected_quote) # this will return undefined
except NameError:
print("selected_quote is not defined outside of getRandomQuote")
# But... since I have the function return a value, I can either save the returned value to a variable
# or (in this case) I can pass the getRandomQuote function as an argument to the print function
quote = getRandomQuote(pixar_movie_quotes) # this statement stores the selected_quote into the quote variable
print(quote) # output will be the value of the selected_quote variable
print(getRandomQuote(pixar_move_quotes)) # output will be the value of the selected_quote variable
I decided to write different code from yours so as to not rob you of figuring out your own solution to the issue you're faced with. Hopefully, what I've written will help to offer you insight into your own code.
If you end up needing a bit more assistance, feel free to reach back out.
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsItβs not really possible to stipulate the reason for your indent error without being able to see how your code is indented, but in order to have it run, it needs to have a consistent indention (the above code Iβve written has each nested layer indented by four spaces).
Four spaces is the typical convention in Python, as such your code should resemble the above to not receive any errors.