Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial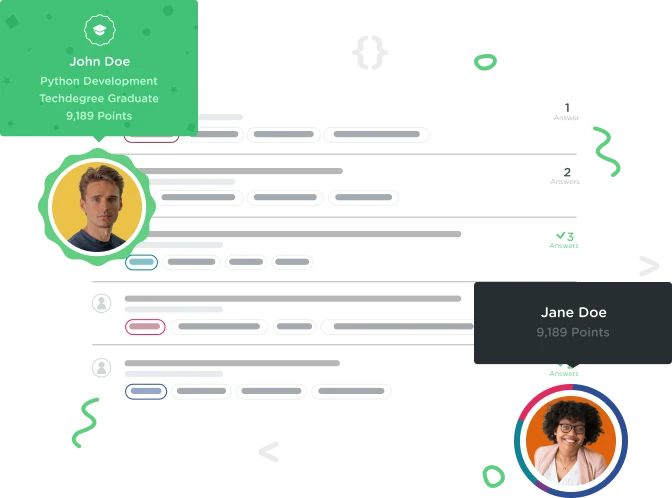

keith tellis
1,065 PointsHaving trouble with this method. Return a count of letters in the tiles.
Can not get the code to work.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter) {
int letterCount = 0;
for (char tile : tiles.toCharArray()) {
if (tiles.indexOf(letter) != -1) {
letterCount++; }
return letterCount;}
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
1 Answer

andren
28,558 PointsThe problem with your code logic wise is that let's say that there are 5 tiles in the tiles
array and the letter
passed in is in fact present somewhere in the array. With your code that would result in the letterCount
equaling 5 after the loop, regardless of whether there actually are 5 instances of said tile.
This is due to the fact that in your loop you are not checking if the individual tiles in the array are equal to the passed in letter
. You are just checking if it is present at all anywhere in the array. And you are doing this one time for each tile in the array.
The point of a for
loop like you are using is that it automatically pulls out the individual items you need to compare without you having to do anything too complicated yourself. The individual item will just exist within the loop within the tile
variable.
If you ever write a for
loop where you don't actually use the variable the for
loop generates that's a pretty good indication that you are doing something incorrectly, as you are ignoring the key element that makes for
loops useful.
Anyway the code you need within the for
loop for this task is actually pretty simple. Simpler than the code you have at present in fact. All you need to do is compare the individual tiles from the tile array (which you have access to in the tile
variable) to the answer
variable. And then increasing the count if they equal each other.
It's also worth mentioning that your for
loop is missing an ending bracket } which is also part of why your code is not passing.
Taking all of that into account you end up with this code:
public int getCountOfLetter(char letter) {
int letterCount = 0;
for (char tile : tiles.toCharArray()) {
if (tile == letter) {
letterCount++;
}
}
return letterCount;
}
Edit: Accidentally pasted the wrong code.