Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial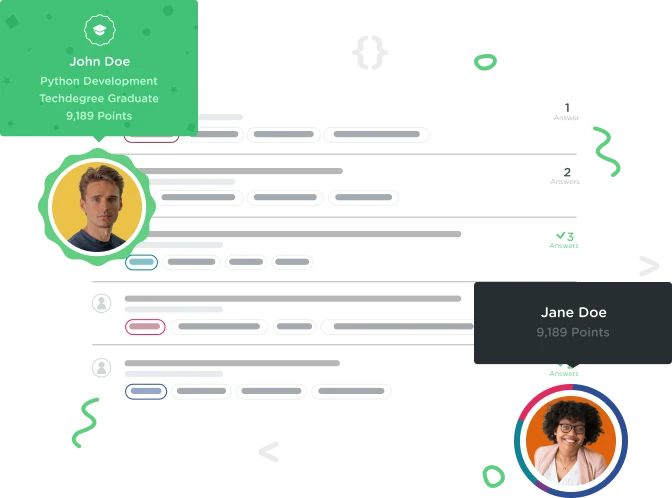
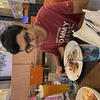
Arnav Goyal
1,167 PointsHelp in exception handling in python !
Hi, the purpose is to prevent the user from entering anything other than a yes or a no. I tried to raise an error if the user doesn't do so and inform them. Can you help in revising this code so it works? Thanks.
name = input("What's your name? ")
if answer != "yes" or "no":
raise ValueError("""Invalid response. Please use 'yes' or 'no' only!""")
try:
answer = input("Hi {}, do you understand while loops?\n(yes/no)".format(name))
answer = answer.lower()
except ValueError as err:
print("{}".format(err))
else:
while answer == "no":
print("Ok {}, while loops in python repeat as long as a certain boolean condition is met.".format(name))
answer = input("Hi {}, do you understand while loops?\n(yes/no)".format(name))
answer = answer.lower()
print("Glad you understood while loops! It was getting repetitive!")
3 Answers
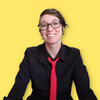
Mel Rumsey
Treehouse ModeratorHey Arnav Goyal
First, you want to take in the input for answer. Then you can raise a ValueError if the answer
does not equal yes or no. (Note: we want the ValueError to be caught, so you will want to raise it in the try
block.
You will also need to write that conditional differently though because it checks for true false. Think of it in this way. if (answer != "yes") or ("no"). The first one checks if the string "yes" is not the same as answer. This will evaluate to True
or False
. But checking if "no"
will always evaluate to True
because a string that is not empty is truthy
.
Take a look at this format and see if that works for you!
try:
answer = input("Hi {}, do you understand while loops?\n(yes/no)".format(name)).lower()
if answer != "yes" or answer != "no":
raise ValueError("""Invalid response. Please use 'yes' or 'no' only!""")
Hope this helps :)
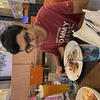
Arnav Goyal
1,167 PointsI understand the conditional statement(silly mistake on my part). I didn't know that you could raise an error in the try block itself. I have made the above changes but the code still doesn't work properly.
name = input("What's your name? ")
try:
answer = input("Hi {}, do you understand while loops?\n(yes/no)".format(name))
answer = answer.lower()
if answer != "yes" or answer!="no":
raise ValueError("""Invalid response. Please use 'yes' or 'no' only!""")
except ValueError as err:
print("{}".format(err))
else:
while answer == "no":
print("Ok {}, while loops in python repeat as long as a certain boolean condition is met.".format(name))
answer = input("Hi {}, do you understand while loops?\n(yes/no)".format(name))
answer = answer.lower()
print("Glad you understood while loops! It was getting repetitive!")
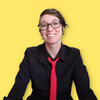
Mel Rumsey
Treehouse ModeratorAh I see. You'll want to make sure that your try/except/else
is wrapped in a while
loop if you want it to run over and over again :)